This post tells you how you can easily make an Android application to extract the text from the image being captured by the camera of your Android phone! We’ll be using a fork of Tesseract Android Tools by Robert Theis called Tess Two. They are based on the Tesseract OCR Engine (mainly maintained by Google) and Leptonica image processing libraries.
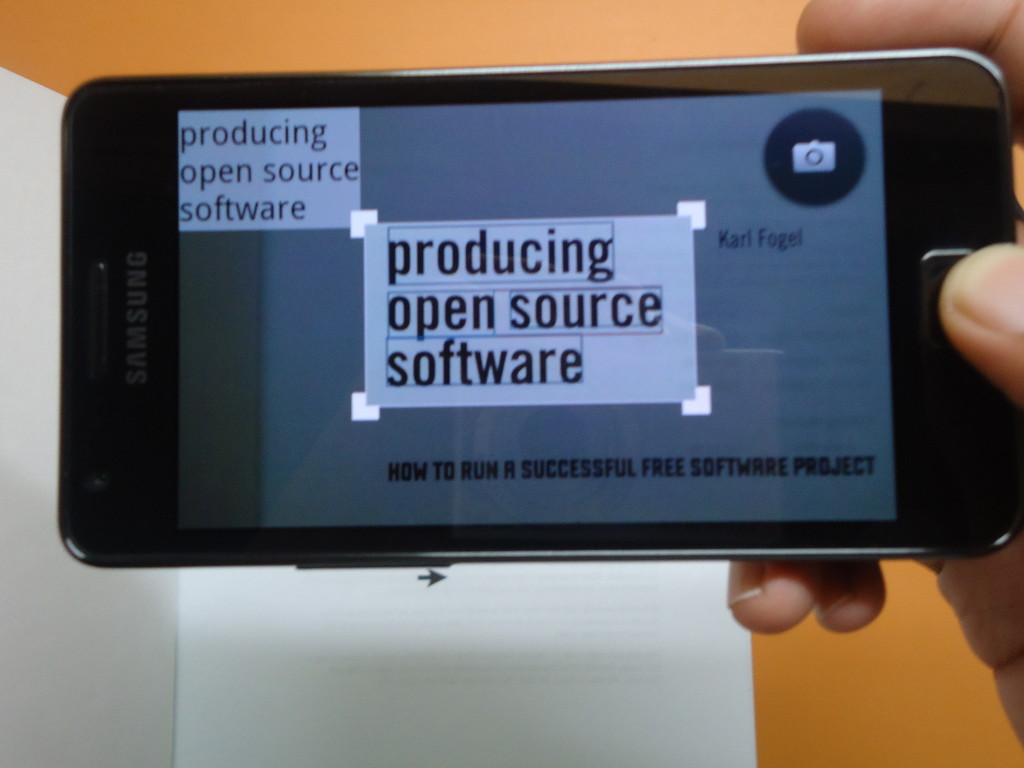
Note: These instructions are for Android SDK r19 and Android NDK r7c, at least for the time being (written at this tree). On 64-bit Ubuntu, you may need to install the ia32-libs 32-bit compatibility library. You would also need proper PATH variables added (see Troubleshooting section below).
- Download the source or clone this git repository. This project contains tools for compiling the Tesseract, Leptonica, and JPEG libraries for use on Android. It contains an Eclipse Android library project that provides a Java API for accessing natively-compiled Tesseract and Leptonica APIs. You don’t need eyes-two code, you can do without it.
- Build this project using these commands (here, tess-two is the directory inside tess-two – the one at the same level as of tess-two-test):
cd <project-directory>/tess-two ndk-build android update project --path . ant release
- Now import the project as a library in Eclipse. File → Import → Existing Projects into workspace → tess-two directory. Right click the project, Android Tools → Fix Project Properties. Right click → Properties → Android → Check Is Library.
- Configure your project to use the tess-two project as a library project: Right click your project name → Properties → Android → Library → Add, and choose tess-two. You’re now ready to OCR any image using the library.
- First, we need to get the picture itself. For that, I found a simple code to capture the image here. After we have the bitmap, we just need to perform the OCR which is relatively easy. Be sure to correct the rotation and image type by doing something like:
// _path = path to the image to be OCRed ExifInterface exif = new ExifInterface(_path); int exifOrientation = exif.getAttributeInt( ExifInterface.TAG_ORIENTATION, ExifInterface.ORIENTATION_NORMAL); int rotate = 0; switch (exifOrientation) { case ExifInterface.ORIENTATION_ROTATE_90: rotate = 90; break; case ExifInterface.ORIENTATION_ROTATE_180: rotate = 180; break; case ExifInterface.ORIENTATION_ROTATE_270: rotate = 270; break; } if (rotate != 0) { int w = bitmap.getWidth(); int h = bitmap.getHeight(); // Setting pre rotate Matrix mtx = new Matrix(); mtx.preRotate(rotate); // Rotating Bitmap & convert to ARGB_8888, required by tess bitmap = Bitmap.createBitmap(bitmap, 0, 0, w, h, mtx, false); } bitmap = bitmap.copy(Bitmap.Config.ARGB_8888, true);
- Now we have the image in the bitmap, and we can simply use the TessBaseAPI to run the OCR like:
TessBaseAPI baseApi = new TessBaseAPI(); // DATA_PATH = Path to the storage // lang = for which the language data exists, usually "eng" baseApi.init(DATA_PATH, lang); // Eg. baseApi.init("/mnt/sdcard/tesseract/tessdata/eng.traineddata", "eng"); baseApi.setImage(bitmap); String recognizedText = baseApi.getUTF8Text(); baseApi.end();
(You can download the language files from here and put them in a directory on your device – manually or by code)
- Now that you’ve got the OCRed text in the variable recognizedText, you can do pretty much anything with it – translate, search, anything! ps. You can add various language support by having a preference and then downloading the required language data file from here. You might even put them in the assets folder and copy them to the SD card on start.
To make things easy, and for you to have a better understanding, I have uploaded a simple application on OCR that makes use of Tess Two on Github called Simple Android OCR (for beginners). If you want a full-fledged application, that has a selectable region while capturing the image, translating the text, preferences etc., then you can checkout Robert Theis’ Android OCR application (for intermediate+)!
Updated: 7 October 2012
References
- Using Tesseract Tools for Android to Create a Basic OCR App by Robert Theis
- Simple Android Photo Capture by MakeMachine
- tess-two README
Troubleshooting
- If you are using Android Studio, check this comment.
- About updating PATH – You need to update your PATH variable for the commands to function, otherwise you would see a command not found error. For Android SDK, add the location of the SDK’s tools and platform-tools directories to your PATH environment variable. For Android NDK, use the same process to add the android-ndk directory to the PATH variable.
- Maven-ising – Check this post by James Elsey. He also mentions that he got it working on Windows without any problems.
- On Windows: “xcopy is not recognized.” Solution: Move xcopy.exe file from Windows\System32 folder to android-sdk\tools folder (or add %SystemRoot%\system32 to the PATH variable).
- On Windows: “The project either has no target set or the target is invalid. Please provide a –target to the ‘android.bat update’ command.” Solution: Run the command
android update project --path D:\Softwares\Studies\Android\
OCR\Code_Project\tess-two- master\tess-two --target android-19 - You may also try Ctrl+F-ing your problem on this page, someone might have already encountered it and posted a solution in the comments.
Translations
- Japanese by datsuns
Projects Made By Users
People have made a lot of projects using this tutorial, some of them are:
- DatumDroid by Aviral, Devashish and me
- MachineRetina by Salman Gadit
- OCR Quickly by UrySoft
If you have made one too, do tell us in the comments below!
Hi, I’m a student and I have a school project. I’m going to make android OCR for Korean Character Recognition. I would like to use tesseract android open source library. I have googled a lot how to build the libjpeg.so, liblept.so and libtess.so from the following Tesseract android readme file. I tried on cygwin and ubuntu, but both of them ended up with some errors. While using Cygwin it said that no rule to make target, while using Ubuntu I succeeded in building libjpeg.so but while building liblept.so errors occurred which said that android/bitmap.h does not exist. Then I check my leptonica-1.68 folder, true there is no such folder and file. I downloaded bitmap.h from internet and put it under android folder but still the same problem. Can u help me out of this ? The deadline will be 1 month and 30 days ahead for me to finish my project and the will be judgement day.
Thank You for your attention
Regards,
Priska
I received the same error and solved it by editing tess-two/jni/Android.mk (as highlighted in step 2) so that the beginning looks something like:
# NOTE: You must set these variables to their respective source paths before
# compiling. For example, set LEPTONICA_PATH to the directory containing
# the Leptonica configure file and source folders. Directories must be
# root-relative, e.g. TESSERACT_PATH := /home/username/tesseract-3.00
#
# To set the variables, you can run the following shell commands:
# export TESSERACT_PATH=path-to-tesseract
# export LEPTONICA_PATH=path-to-leptonica
# export LIBJPEG_PATH=path-to-libjpeg
#
# Or you can fill out and uncomment the following definitions:
# TESSERACT_PATH := path-to-tesseract
# LEPTONICA_PATH := path-to-leptonica
# LIBJPEG_PATH := path-to-libjpeg
TESSERACT_PATH := $(call my-dir)/../external/tesseract-3.01
LEPTONICA_PATH := $(call my-dir)/../external/leptonica-1.68
LIBJPEG_PATH := $(call my-dir)/../external/libjpeg
You can also try running these in the Terminal before ndk-build in step 3:
export TESSERACT_PATH=${PWD}/external/tesseract-3.01
export LEPTONICA_PATH=${PWD}/external/leptonica-1.68
export LIBJPEG_PATH=${PWD}/external/libjpeg
Hope that it helps. 🙂
Where to put the google translate API key & bing translator Client ID and Client Secret for translation?
Hi Gautam, Thanks alot for such a helpful post. I am also an indian and doing my masters in augmented reality in Malaysia. Really proud to see our young indian blood like you solving out the worlds problems 🙂 God Bless you….keep up the good work brother.
Salam/Peace
Hello, I made the whole process and the project gives me tess-two errors:
Requires Android compiler compliance level 5.0 or 6.0. Found ‘1 .4 ‘instead.
I compiled with JRE 1.5, this is okay?
Thanks in advance, greetings.
Yes, that should be okay. Just do Fix Android Properties if there’s anything. 🙂
Thanks!! I tried it and it is working ..
Where can I get a library where an image is filtered to only get the blacks?, Ie., Eliminate funding of an image and be alone with the text to parse the OCR system
What I do is have for example this picture
http://www.juegosonce.com/dicadiw/img/SuperOnce_6.jpg
Getting an image by extracting only the text on the front and delete text and background colors.
Is there any library in Android for this?
None that I particularly know of, but you may check this post for more information – http://www.bitquill.net/blog/?p=119
https://play.google.com/store/apps/details?id=com.davecote.seesaytranslate
Travel with See Say! Image to Speech translator, take a picture and translate!
An app for android that takes a picture, and speaks the text aloud, in any language:)
I was inspired by a Ted Talk that mentioned that not too long ago, this technology was available for around $10,000.00, for the visually impaired. Technology has come so far that I am able to write this app and release it for $1.99 🙂
Similar to See Say – Picture to Speech, but the image recognition will translate from other languages to English.
Also, gives option of Speech or Text, in case you don’t want your phone to speak (ie: in a university lecture:)
GREAT for traveling! Imagine you are in a country and don’t understand the language, you can take a picture of a menu at a restaurant, and the items are read back to you in English!
Enjoy!
Oops I forgot to mention, I’ve posted the code, open source, in the description on Google Play:)
I need your help for the android ocr app I am making as my project. I did all the steps successfully. I then imported your project along with tess-two in eclipse. It shows no errors, but it fails at run time. I really made a lot of error to sort it out. It’ll be great if you can be of any help.Contact me on my email id. I’ll be obliged.
Check logcat. That should show why it’s failing.
It tapered down to:
Could not find tess-two.apk!
Hey Gautam,
I tried following the steps listed by you in windows environment (using cygwin). However, I face this error after I do ndk-build:
make: *** No rule to make target `//cygdrive/f/work/ocr_cc/newf/rmtheis-tess-two-1edb5e2/rmtheis-tess-two-1edb5e2/tess-two/external/leptonica-1.68/src/adaptmap.c', needed by `/cygdrive/f/work/ocr_cc/newf/rmtheis-tess-two-1edb5e2/rmtheis-tess-two-1edb5e2/tess-two/obj/local/armeabi/objs/lept//cygdrive/f/work/ocr_cc/newf/rmtheis-tess-two-1edb5e2/rmtheis-tess-two-1edb5e2/tess-two/external/leptonica-1.68/src/adaptmap.o'. Stop.
I tried solutions mentioned at http://code.google.com/p/tesseract-android-tools/issues/detail?id=4#c16 but with no luck.
I strongly suspect that there is a path problem because if you look at the path in the error, it looks like it is a absolute path problem. Can you suggest any work around?
Thanks,
Vishwanath
That’s the same problem that was being faced by one of my friends, I tried to fix it via Teamviewer but couldn’t. I’d prefer if you develop on non-win system or contact Robert Theis, author of tess-two for help. 🙂
thanks gautam. switched to linux! 🙂
Hi,
Get error during build :
utilities.cpp:19:28: error: android/bitmap.h: No such file or directory
any idea ?
tx
ps
the tesseract-android-tools are working for me
Have you edited the Android.mk file as explained in comment 6611?
Yes,I did all steps again , clone ,uncoment lines (12-18)in Android.mk and build . Get same error :
Compile++ thumb : lept <= utilities.cpp
tess-two/tess-two/tess-two/jni/com_googlecode_leptonica_android/utilities.cpp:19:28: error: android/bitmap.h: No such file or directory
make: *** [tess-two/tess-two/tess-two/obj/local/armeabi/objs/lept//tess-two/tess-two/tess-two/jni/com_googlecode_leptonica_android/utilities.o] Fehler 1
Which OS do you use? I just built the latest revision on my Mac without any problems.
hi andrzej,
I had the same problem as yours.
Did u extract the android-ndk from terminal?
I also extracted the file from terminal, but some file was missing. So I downloaded and installed the new android-ndk and extracted it by clicking the right mouse instead of using terminal.
I suggest you to reinstall your android-ndk to the latest version. android/bitmap.h is included in android-ndk.
hi,
@priska , thanx for suggestion ,but the problem was building with NDK r6 .
Now NDK r6b is building without any error, so this problem is solved.
The other problem was by android update :
android update project --path .
Error: The project either has no target set or the target is invalid.
Please provide a --target to the 'android update' command.
For any one having this error : update your Android SDK Tools to r14/r15
ubuntu 10.04
I can build tesseract-android-tools without any problems.
Dont understand what wrong 🙁
I am waiting for some reply also here:
http://code.google.com/p/tesseract-android-tools/issues/detail?id=9
thanx
Which version of the SDK Tools and NDK are you using?
@rmtheis, yes the version was a reason of errors. Is solved, see my other Comment.
make: *** No rule to make target `//home/park/android-ndk-r7/App/tess-two/external/leptonica-1.68/src/adaptmap.c', needed by `obj/local/armeabi/objs/lept//home/park/android-ndk-r7/App/tess-two/external/leptonica-1.68/src/adaptmap.o'. Stop.
error… help me
It’s not working with NDK r7 at the moment. Use NDK r6b.
I think there should be path problems: “//home/park/android-ndk-r7/” and “/lept//home/park/” which are invalid paths.
Please help i got this error.
c:\rmtheis-tess-two-1edb5e2\tess-two>c:\MyWork\android-ndk\ndk-build
Install : libjpeg.so => libs/armeabi/libjpeg.so
"Compile thumb : lept <= open_memstream.c
"Compile thumb : lept <= fopencookie.c
"Compile thumb : lept <= fmemopen.c
"Compile++ thumb : lept <= box.cpp
In file included from jni/com_googlecode_leptonica_android/box.cpp:17:
jni/com_googlecode_leptonica_android/common.h:22:24: error: allheaders.h: No suc
h file or directory
jni/com_googlecode_leptonica_android/box.cpp: In function 'jint Java_com_googlec
ode_leptonica_android_Box_nativeCreate(JNIEnv*, _jclass*, jint, jint, jint, jint
)':
jni/com_googlecode_leptonica_android/box.cpp:27: error: 'BOX' was not declared i
n this scope
...
make: *** [obj/local/armeabi/objs/lept/box.o] Error 1
The box.cpp it not correct? any build source work please send to my mail.
The build doesn’t work on Windows as far as I know.
hmmm i can build on windows it generate those .so file but when test on my phone got error 2 more:
1. NativeReadBitMap(ReadFile.java:203)
2. SetImage(TessBaseAPI.Java:311)
How to solve it problem 😀
Nope, the fact that the files were created doesn’t necessarily mean the build worked. Give it a try on Linux.
I made it following this post!!! The Simple Android OCR is a really cool app! I am playing it on my Nexus One now. During the whole process, I did not encounter any problem. Built it under Ubuntu 10.04 64bit.
Hi , any one has some good source/Dok/links how to work with implemented functions of tesseract?
How to use :
api.setPageSegMode(mode)
api.setRectangle(rect)
api.getTextlines()
also
Binarize.otsuAdaptiveThreshold(pix);
Binarize is important by ocr ,but I am getting no result … or I don’t know how to use it…
That method returns a Pix object, so you would need to do:
Pix myThresholdedImage = Binarize.otsuAdaptiveThreshold(pix);
i am getting error of TessbaseApi .and its giving me error that googlecode can not be resolved to a type?
use this in your gradle
dependencies {
compile ‘com.rmtheis:tess-two:5.4.1’
}
thanks for this superb tutorial Gautam. It worked for me after couple of messy configurations on my Fedora boot. Wish you all the best for your future endeavors.
Is it just me or is the text recognition really poor using the tesseract API? I tried this and most of what I got was garbage (picture taken with 8MP HTC Evo camera, moderately good light conditions, printed text black on white). Even with rmtheiss’ Android OCR app, I got very poor results, plus its pretty slow. Uploading the same image to Google Docs and using its OCR had way better results. I’ve been thinking about trying to use the Google Docs API to do OCR for me but not sure that’s a good idea, considering it uploads the file as a new google doc and that’s not what I want, I just need to be able to OCR it and then parse the text for key info, not save it. I could set up my own server for cloud OCR-ing but I see no point in that unless I can get better OCR results. Any suggestions on how to make the recognition better?
Did you try the intermediate+ project?
That project gave much better results. Currently, I’m not exactly sure why though. Perhaps, it’s because it allows the user to define an area of interest.
hi Gautam.
Thank you for amazing post !!
I had tried to build Tesseract-Android, and never succeeded until I found this site!
and I translated this information into Japanese on my blog.
http://d.hatena.ne.jp/datsuns/20120105
(sorry Japanese only…)
I’m sure this will be good information for other Japanese engineers!!
so many Thanks !!
Thanks for that. 🙂
Hii, i tried this sample and i am getting dalvikvm: Exception Ljava/lang/UnsatisfiedLinkError; i am using windows xp, and i complied to get lib file using cygwin. The application runs up to image capturing after that i am getting force close error. can you pls help me where i am getting struck off. i am new to ndk and ocr integration.
It looks like it doesn’t work on Windows even using Cygwin. Can you try doing it on Ubuntu VM?
Hii, no i only tried on Windows, whats the alternative solution for this.. is there any source file available for ready to use.??
Sorry, but this doesn’t work on Windows as noted in the previous comments and the post. 🙂
i am facing the same error although i am using a mac.
I’ve been looking into getting a live camera preview working in the Android emulator. Currently the Android emulator just gives a black and white chess board animation. ..plz help me ?
That’s the intended behavior–the chess board animation simulates a camera view.
There’s some code online that you can try searching for that connects the video feed from your webcam to the camera input of the Android emulator. It will probably be somewhat challenging to set up, but I’ve heard that it works.
dear Gautam
i have a problem at step number 3 and error message said “ndk-build:command not found”
please help me fix this thing
thank you
You need to add the android ndk directory to your PATH variable as noted in the post. 🙂
how do i add NDK directory to my PATH variable? i didn’t find note ini the post
sorry im really newbie with this thing
hi Gautam
i have succeessfully passed the “ndk-build” step and advanced to the “android update project –path .” step but it didn’t work and error message said “android: command not found”
what should i do to solve this problem
thank you
me too.
i passed the “ndk-build” step.
but when i try “android update project –path .” step, it didn’t work and error message said “android: command not found”
You need to add /…path…/android-sdk/tools/ to your PATH variables, which contains the android command.
I’ve now added About updating PATH section in Troubleshooting. Please check it. 🙂
Hey, your work is great. 😉
hi Gautam
i have problem again. when i tried to add library as the instruction number 5, there is nothing tess-two library. i think i missed something.
please help me fix this thing
thank you Gautam
You need to import the tess-two project as a library in Eclipse as stated in step 4.
Thank you!!
it works great !!
Keep it up…!!
I’m working on MAC and I have Android SDK latest installed and got the NDK r6 butwhen I use the
ndk-build I get the follwoing error whe it is trying to build the lept lib:
Invalid attribute name:
package
Install : libjpeg.so => libs/armeabi/libjpeg.so
SharedLibrary : liblept.so
/Users/viph4367/android-ndk-r6/toolchains/arm-linux-androideabi-4.4.3/prebuilt/darwin-x86/bin/../lib/gcc/arm-linux-androideabi/4.4.3/../../../../arm-linux-androideabi/bin/ld: cannot find -ljnigraphics
collect2: ld returned 1 exit status
make: *** [/Users/viph4367/Tesseract/obj/local/armeabi/liblept.so] Error 1
Any idea, what I may be missing or doing wrong here?
Vijay
Update:
– downloaded Robert Tess-two
– and used NDK r7 as he mentioned in his README
– I still get an error while running ndk-build, but a differnet on:
make: *** No rule to make target …/rmtheis-tess-two-0cddf3a/tess-two/jni/../external/leptonica-1.68/src/adaptmap.c’
Any ideas??
Latest Update:
Got it working. My build term had some issues. I got a new term which solved the issues I was running into.
Vijay
i tried building using ndk-build but it gives the follwing error.
Install : libjpeg.so => libs/armeabi/libjpeg.so
make: *** No rule to make target `/home/sumit/Downloads/rmtheis-tess-two-0cddf3a/tess-two/jni/com_googlecode_leptonica_android/../..//home/sumit/Downloads/rmtheis-tess-two-0cddf3a/tess-two/external/leptonica-1.68/src/adaptmap.c', needed by `/home/sumit/Downloads/rmtheis-tess-two-0cddf3a/tess-two/obj/local/armeabi/objs/lept//home/sumit/Downloads/rmtheis-tess-two-0cddf3a/tess-two/external/leptonica-1.68/src/adaptmap.o'. Stop.
I working with Ubuntu
Thanks in advance!!
Be sure to use SDK r16 and NDK r7. Get the latest tess-two.
Thanks for your reply Vijay. I have been using SDK r16 and NDK r7. I also got a fresh copyof tess-two. Nothing seems to be working. I also tried with NDKr5. Still no luck!!!
I have the same problem with you. I believe they changed the code since my last compiling in early Dec 2011. Because I can compile my old cold successfully again but fail in the code downloaded from the repository today. The path is broken.
I think maybe tess-two changed some path variables in January 04, 2012 when they tried to integrated the eyes-two code into the project. “com_googlecode_leptonica_android/../..//home/sumit/” and “/lept//home/sumit/” in the error message really look like a path setting problem. (“//” before home)
how can i get a working copy of tess-two code?
I not sure how many people encounter this problem as us. It seems many people work well with the new release.
I can’t reproduce this problem–I just pulled from the repository and it built successfully on NDK r7 and Android SDK Tools 16 on Ubuntu 11.04 by following the instructions here.
Please let us know if you find the source of the problem.
OK it looks like this problem is related to Ubuntu 11.10. Until I get a chance to fix it, try building on 11.04.
I’ve updated the post to reflect the changes that it now works with SDK r16 and NDK r7. Also, it doesn’t require all those TESSERACT_PATH, LEPTONICA_PATH and LIBJPEG_PATH. 🙂
I get this problem:
android update project –path .
Error: The project either has no target set or the target is invalid.
Please provide a –target to the ‘android update’ command.
When using Android SDK Tools r15. I’m currently upgrading to r16 and will try again.
Updating to r16 resolved the issue! Hurray! Thanks for putting this together.
Thanks for your great post, Guatam.
It helped me a lot and the examples work great too.
I have a question.
Is it possible to make ‘Tesseract’ recognize more than two languages at the same time?
It seems to be that ‘Tesseract api’ is only initialized with one language even if I copy more language data files to sdcard.
Any advice will be really appreciated.
Thanks and have a nice day!!
I don’t think that is possible currently. You may want to run the OCR multiple times, using different languages each time and put the strings together. 🙂
Thank u so much for ur kind answer Gautam!! At least I’m now aware of what I need to do! Best luck for you:D
Hey gatuam,
I am working on OCR project and I came across your blog.
I have been finding some difficulty in building
android-NDK in MAC OSX LION, I downloaded its latest version r7 as you have mentioned, but when I give the path for ndk-build, it gives error as
“ERROR: Cannot find ‘make’ program. Please install Cygwin make package or define the GNUMAKE variable to point to it.”
There are no spaces in my ndk path also, It would be great if you help us out.
Thanks
Please Google that error, you would find some good answers to resolve the problem. 🙂
1.I complie in cygwin follow the readm steps..
2. and import in my project(myocr) as library project.
3. now when i try to run the program my application myocr.apk goes in android emulator and install but when the tes-two project library turns come it display the error that
“Could not find tess-two.apk!”
🙁
Please Help
Asad, as mentioned earlier in the post and the comments, it does not work on Windows even with Cygwin. Please try using an Ubuntu VM. 🙂
1. Is (my android library project) tess-two.apk also install on my android emulator yes or not ?
If Yes then first time when i set up and run my ocr project this tess-two.apk also install in android, but after 4 or 5 times i dont know what i did wrong in my project settings it is not installing give error Could not find tess-two.apk!”
2. The Tess-two Project was build using API 3.2 i changed it to android API 2.2 is it ok to change the API level ?
cuz m using emulator in android API Level 2.2.
s@ubuntu:~/Desktop/android-ndk-r7$ ./ndk-build NDK-LOG=1
Android NDK: Your APP_BUILD_SCRIPT points to an unknown file: home/s/Desktop/tess-two/jni/Android.mk
/home/s/Desktop/android-ndk-r7/build/core/add-application.mk:133: *** Android NDK: Aborting... . Stop.
we are using ubuntu 11.04 on VMware.Android.mk file is present in the required folder.Also we have tried it on android-ndk-r6b but the same error persists.Awaiting a solution.plz.
Does it also fail when you run ndk-build from the “tess/tess-two” project directory (as specified in the instructions)?
Hi Gautam
i have downloaded the application’s source code that you made. i created a new project then used the code and then run it. i have been successful deploying this project to the android device. i run the application on the device, using camera to complete the action. i captured the picture and then process stopped unexpectedly.
i found a couple of error messages on eclipse that refer to some of code lines
1. line 211 at SimpleAndroidOCRActivity.java refers to this line of code :
TessBaseAPI baseApi = new TessBaseAPI();
2. line 35 at SimpleAndroidOCRActivity.java refers to this line of code :
onPhotoTaken();
3. line 47 at TessBaseAPI.java refers to this line of code :
System.loadLibrary(“lept”);
Are you sure that you’ve added the Tess library to the application project (step 4)?
yes i have…therefore error on TessBaseAPI.java at line 47 appeared
i think the errors related to TessBaseAPI.java, especially at line 47, it couldn’t load “lept”
“3. line 47 at TessBaseAPI.java refers to this line of code :
System.loadLibrary(“lept”);”
Did you solve the problem? I get the same error.
i found the same problem….please check this link:
https://github.com/rmtheis/tess-two/issues/4
the error messages exactly same as i got
i’ve an issue:
the program failed at “Before baseApi” LOG.
W/dalvikvm(11691): Exception Ljava/lang/UnsatisfiedLinkError; thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI;
this is the error.
how can i solve it?
when i type the command ndk-build it gives following error
install : libjpeg.so => libs/armeabi/libjpeg.so
make: *** No rule to make target `/home/kashif/tess/tess-two/jni/com_googlecode_leptonica_android/../..//home/kashif/tess/tess-two/jni/../external/leptonica-1.68/src/adaptmap.c’, needed by `/home/kashif/tess/tess-two/obj/local/armeabi/objs/lept//home/kashif/tess/tess-two/jni/../external/leptonica-1.68/src/adaptmap.o’. Stop.
and after android update project --path
and ant release
its sucessfully build.xml file. and when compile project to add library with simple android ocr then display error in logcat as follow
02-17 17:42:57.369: E/AndroidRuntime(330): FATAL EXCEPTION: main
02-17 17:42:57.369: E/AndroidRuntime(330): java.lang.ExceptionInInitializerError
02-17 17:42:57.369: E/AndroidRuntime(330): Caused by: java.lang.UnsatisfiedLinkError: Couldn’t load lept: findLibrary returned null
please help me what i do i am totally stuck from 2 and half week.
You need to use Ubuntu 11.04 instead of 11.10.
I’m not sure if Ubuntu 10.04/10.10 work.
Just to let everyone know – just built tess on Windows 7 today 😉 I didn’t do anything special, only steps described in this article – no errors so far. Tomorrow I will try to configure tess in eclipse.
Hi, i’m see in your comment that you’l try to configure tess on eclipse. I am trying too, but with no sucess. You did it? How? Please, i’m trying to find tutorials about and nothing concret until today.
@Gautam and @rmtheis, i have a suggestion to you. Why not you record video on how to compile those things. from scratch to the end. because this project are really2x important. many of newbies wanna try to their own projects. i need you help on this. could you please do that?
really appreciate.
I’ve found some video tutorials to be really helpful (for example, this one on Git/GitHub: http://www.youtube.com/watch?v=TPY8UwlTIc0), but I don’t think this topic is well suited for video format, so I don’t have plans to make a video.
Hey,
I successfully compiled tess-two and completed the steps up until 3, however when I tried to set it as a library in eclipse it wouldn’t save the “is library” setting. Anyone have a similar problem or a possible solution?
Thanks
That’s weird. You could try adding
android.library=true
to the project.properties file.when I run ndk-build, it fails complaining from the following errors:
$ ndk-build
make: /.../android-ndk-r7/toolchains/arm-linux-androideabi-4.4.3/prebuilt/linux-x86/bin/arm-linux-androideabi-gcc: Command not found
Compile arm : jpeg <= jcapimin.c
make: /.../android-ndk-r7/toolchains/arm-linux-androideabi-4.4.3/prebuilt/linux-x86/bin/arm-linux-androideabi-gcc: Command not found
make: *** [obj/local/armeabi/objs/jpeg/jcapimin.o] Error 127
The problem is that although it says "command not found", "arm-linux-androideabi-gcc" exists in the above path. Even when I run "arm-linux-androideabi-gcc" directly from /…/toolchains/arm-linux-androideabi-4.4.3/prebuilt/linux-x86/bin/ it gives the same error of "command not found"
I also added ./toolchains/arm-linux-androideabi-4.4.3/prebuilt/linux-x86/bin to my PATH but still getting the same error. Even I tried different versions of ndk (7, 7b, 6b) still same error! seems to me the above gcc is meant for 32bit machines whereas my machine is "Linux 2.6.32-37-server x86_64". but I guess the ndk package should work for both 32 and 64bit. am I right? do I need to compile or build ndk before using it? I assume downloading and unpacking is all I have to do. right? how about sdk? I just downloaded and unpacked it at the same folder that I have my ndk. do I need to configure them to work with each other?
It was the problem of machine. I tried it on Ubuntu 11.10 and everything worked perfectly!
Hey Gautam,
Currently I am trying to get tesseract android tools
http://code.google.com/p/tesseract-android-tools/
to work for me on Android. I have been going at this for about a week to no avail.
I am running Win 7 64 bit with cygwin.
I followed the instructions in the readme file and made many changes to the Android.mk files. Basically it was appending a slash to the path, so I had to manually hard code the paths of the individual files, or move to location of the files within the 3 packages to get it to build. However at the end of the build, I did not recieve a “Build Sucessful” notice, but the .so files were generated.
I ported it to eclipse as is and used your code to get the extracted text.
private static final String TESSBASE_PATH = "/mnt/sdcard/";
Bitmap imageFile = BitmapFactory.decodeFile(image.getAbsolutePath());
TessBaseAPI baseApi = new TessBaseAPI();
if(baseApi.init(TESSBASE_PATH, "eng")){
System.out.println("Tessbase initialized");
baseApi.setDebug(true);
baseApi.setImage(bmp);
String recognizedText = baseApi.getUTF8Text();
System.out.println("---------------------output-------------------");
System.out.println("recognizedText");
}
else{
System.out.println("Tessbase initialization failure.");
}
At first I was getting an error saying
“Bitmap functions not available; library must be compiled under android-8 NDK”
After taking a look at the tessbaseapi.cpp file I realized that it needed a specific compiler flag to compile the correct function. This flag was -DHAS_JNIGRAPHICS. What I think this means is that the JNI Graphics library must be present.
Yet the program still wouldn’t compile because the memcpy() function in the newly compiled method could not be found. I fixed this by changing the actual C++ code to include
Finally the program compiled fully (still wasn’t getting a BUILD SUCCESSFUL notice though) and when I ran it, I did not get any output at all. This could be a problem with the eng.traineddata file, or could be a problem in the actual code.
Is there anything I have done wrong? Can someone link me to and eng.traineddata file that they know works and image that works with it?
Thanks in advance!
Gautam accoring to this page
http://developer.android.com/guide/developing/projects/index.html#LibraryProjects
, the android library project does not contain .apk extension file and it does not install on your device. ok
that was a confusion for me now i fix it, so now i m stuck in this instruction :
baseApi.init(myDir.toString(), “eng”);
my android application install work fine every thing when it comes to this instruction my application crash.
also i tried manually specifying the directory
api.init(“/mnt/sdcard/tesseract/tessdata/”, “eng”);
still error application crash.
and also check in my emulator SD-card no directory are their of tesseract in sdcard.
please tell me what i m doing wrong.
You need to make the application copy the english trained data from its resources folder (you’d have to manually place that in that) to the sdcard on installation/run. You can have a look at that code in the Simple Android OCR application here.
There is a lot of discussion around this only working on Ubuntu.
I’m thinking, once you’ve built it for the first time, could you not just copy it over to windows and never be bothered about Ubuntu again?
Also, can’t the android library project be bundled up so you build it once and just import anywhere else for general usage? Maven might be an option for this.
It may be possible to just distribute the library as a JAR file with the object files added to it.
Releasing the pre-compiled library project on Maven or elsewhere, or tweaking the makefiles so it actually builds on Windows are also workable I think.
Hey gautam i am planning to incorporate OCR in my app for reading pattern from using camera without taking a picture (just like we see in QR reader).i read your article but i couldn’t find anything for windows.i am using windows 7 windows OS and eclipse for developing this app.can u tell me what are the steps for using tesseract ?
Did it finally work on windows 7 + eclipse. How do you go pass the unsatisfied link error?
getting force close after taking photo.error is at TessBaseAPI.please help me.will this project work on ecilpse in windows??
It worked in my application good. But when I changed my application version to 2.3.3 (from 4.0) it gives me error
Android Launch!
[2012-03-05 13:33:36 - fyp2] adb is running normally.
[2012-03-05 13:33:36 - fyp2] Performing nicatoid.fyp2.Fyp2Activity activity launch
[2012-03-05 13:33:36 - fyp2] Automatic Target Mode: Preferred AVD 'my_adv_google' is available on emulator 'emulator-5554'
[2012-03-05 13:33:36 - fyp2] Uploading fyp2.apk onto device 'emulator-5554'
[2012-03-05 13:33:38 - fyp2] Installing fyp2.apk...
[2012-03-05 13:33:43 - fyp2] Success!
[2012-03-05 13:33:43 - fyp2] Project dependency found, installing: tess-two
[2012-03-05 13:33:43 - tess-two] Uploading tess-two.apk onto device 'emulator-5554'
[2012-03-05 13:33:45 - tess-two] Installing tess-two.apk...
[2012-03-05 13:33:46 - tess-two] Installation error: INSTALL_FAILED_OLDER_SDK
[2012-03-05 13:33:46 - tess-two] Please check logcat output for more details.
[2012-03-05 13:33:46 - fyp2] Launch canceled!
How can I fix it? // I changed target sdk to 10 minimumsdk version to 10 but still error occurs.
Thanks.
Can you guide step-by-step how to install NDK? i have refer severals website, still don’t understand.
here it is the official android ndk site
http://developer.android.com/sdk/ndk/index.html#installing
actually you just have to extract it
yes, i have extract it..but i have no idea how to set up the environment. i am referring here : http://rmtheis.wordpress.com/2011/06/02/setting-up-a-virtualbox-ubuntu-development-environment/ , but the statement are too general. i am the beginner. need help.
if you follow step by step instructions from this site actually you don’t need any other NDK setting up process….just extract it
i think step by step instructions from this site are obvious
maybe you shouldn’t combine instructions from few source if you really don’t understand the problem
i suggest you follow the instructions from beginning in this site…i have followed step by step instructions from this site and i have succesfully made OCR application using Tesseract engine
CMIIW
When i install http://subclipse.tigris.org/update_1.6.x , these error occurs. Subversion Native Library not available.
Failed to load JavaHL Library.
These are the errors that were encountered:
no libsvnjavahl-1 in java.library.path
no svnjavahl-1 in java.library.path
no svnjavahl in java.library.path
java.library.path = /usr/lib/jvm/java-6-openjdk/jre/lib/i386/client:/usr/lib/jvm/java-6-openjdk/jre/lib/i386::/usr/java/packages/lib/i386:/usr/lib/jni:/lib:/usr/lib
what actually i am lacking yah?
You don’t need Subclipse to build the project.
i got a problem. when the time i gonna pui-in this command into the terminal, the termina said “couldn’t find package ia32-libs”. i tried googling but still, no luck.
sudo apt-get install vim chromium-browser eclipse-platform libarchive-zip-perl libtext-csv-xs-perl libxml-simple-perl git-core gitosis subversion mercurial ia32-libs conky-all proguard gnupg flex bison gperf build-essential zip curl zlib1g-dev libc6-dev lib32ncurses5-dev x11proto-core-dev libx11-dev lib32readline5-dev lib32z1-dev libgl1-mesa-dev libarchive-zip-perl libfile-find-rule-perl subversion build-essential g++ pkg-config gawk libxml2 libxml2-dev libxml2-utils xsltproc flex automake autoconf libtool libpcre3-dev
Really useful, it works at first attempt.
But it can’t configure it to read only digits. i’ve to OCR .gif images like this one http://goo.gl/9NXrQ and it recognize it as “os/5614360…” i tried with
baseApi.setVariable(“tessedit_char_whitelist”, “0123456789”);
but seems not work, it always recognize starting numbers as “os”/56…
Can you solve me this problem? Many thanks!!!
I’m getting this error…..
The project cannot be built until build path errors are resolved …
what do i do ? let me know the solution
Hello Gautam,
I have two doubts:
1) Can i use Cygwin Terminal in windows for running both leptonica and tesseract? I have read somewhere it do the same.
2)Why i cnt run it in only device containing android 4 os?
Please help me. I am stuck on it.
Thax,
Dhrupal
Hi Gautam ndk-build command was sucessful,but I am facing some problem at android update project –path,I am giving tess-two path but is is sating invalid directory
Sometimes, Cygwin requires full rights to compile…
chmod -R a+rwx tess-two
Would save the day 😀
getting ERROR:
make: *** No rule to make target `/cygdrive/c/Users/JENIL/Downloads/rmtheis-tess-two-071820a/rmtheis-tess-two-071820a/tess-two/jni/com_googlecode_leptonica_android/../..//cygdrive/c/Users/JENIL/Downloads/rmtheis-tess-two-071820a/rmtheis-tess-two-071820a/tess-two/jni/../external/leptonica-1.68/src/gifio.c', needed by `/cygdrive/c/Users/JENIL/Downloads/rmtheis-tess-two-071820a/rmtheis-tess-two-071820a/tess-two/obj/local/armeabi/objs/lept//cygdrive/c/Users/JENIL/Downloads/rmtheis-tess-two-071820a/rmtheis-tess-two-071820a/tess-two/jni/__/external/leptonica-1.68/src/gifio.o'. Stop.
plz suggest me solution…
Hey I am Sayali a final year student of Computer Engineering and as our BE project we are making an OCR android app like yours.We have followed your steps and are working on ubuntu 10.10 but after the ndkbuild step we are getting some random error about adaptmap which we cant sort out. Can you please help us??
Hey Sayali,
I have got the same error. How did you solve the issue? Please help.
Awesome stuff. Helped me a lot in my android project. Thanx Gautam! Keep up the good work! =)
I was able to do this all on windows without any hassle, just followed tutorial and compiled from the command line, so it’s not linux only :S
Results were rather poor, I gave it an image of a car, and instead of spotting the numberplate, it basically tried to create a textual representation of the car itself, like ASCII art..
hey James plz explain how you compile tess-two on windows ?? used cygwin??
hey james help me for ‘ndk-build’ in windows
Thank you..
Gautam,
I have problem android update project –path. I try to click the “About updating PATH” under troubleshooting, but the link not work/come out.
Where to refer? Can you give me step-by-step on this?
Hey JAMES…could you please tell me how to use ndk-build … where to use this command..pls pls pls .. i’m newbie to android platform..
@rmtheis, after i edit the android.library=true to the project.properties file, i successfully cheik Is library box.But, when i want to add tess-two in project selection, there were nothing come out. just a blank. where’s my mistake?
project selection are empty.
ERROR: resource directory '/home/aminalzanki/workspace/tess-two/res' does not exist
Same problem here. The “Is Library” is already checked but when I clicked Add button, the window displays no selection for tess-two library
Thank you for your reply.
HI,
I have downloaded ur sample project and built the ndk also. I cant understand where we are actually implementing the eng.trainnedata from the assets folder. because i dont see any comparison of the image with the buffered trainned-data file.
hey moreover it will be very much helpful if any of u can provide me a method to ocr an image by uploading via sd-card (not via camera)
If you want to compile your code without Linux, you can use [Cygwin] (here) which emulates a BASH (= more or less a Linux Terminal).
Hi Gautam, i’m going to develop an android app that compares two images and displays the name of the picture if the two images are similar. I should compare only the contents of the image, not the pixels. I mean the two images may be in different sizes but they should be same when we compare. How can i achieve this image recognition in android? Can you recommend any open source library which can achieve this in android?
Thanks!
This is not something that Tesseract is meant for, but such technologies do exist. Such content recognition is done by Google Goggles and something similar was accomplished by a friend I met in an event, whom I am no more in touch with. You might find something by Googling more.
@gautam, @emtheis..After i check the Is Library, and want to choose tess-two, the tess-two wont come out. There are just empty. So, i dont know how adding a reference to a library project in the properties of an application project in my case.
many thanks.
Amin.
I am facing the same problem as you. And i am still unable to resolve it. When i click the add button (in the ‘is library’ frame, the entire window is greyed out with only Cancel being active. So i am also stumped there.
AJ
I would like to develop an android app that can recognize handwriting words written on the touch screen of the android phone in real-time(on-line).
How can I do this with tesseract?
This is not something that Tesseract is meant for, but such technologies do exist. You might find them by Googling more. 🙂
Hi Gautam, isn’t binarization of the image required before we make a call to getUTF8Text() ?
Thanks,
Shamy
One more doubt… I have followed the steps you described and I got my app working. But I want to know whether I will able to debug through the Tesseract C++ code and if yes how?
Thanks,
Shamy
I will hope to develop application as your one for my BSC degree. As I read your comments, you have mentioned this is not working in windows. Please can u tell what the changes to run this application on windows.
It may build on Windows (not Cygwin–just Windows) by itself.
If not, you can build tess-two on Linux, transfer the library folder to Windows, and add that folder as a library project in your OCR app.
Is it possible to build the tess-two library in Ubuntu 11.04 (VirtualBox), then transfer the built library into windows and develop an app around it there, or will that not work? I seem to have successfully built tess-two, but when trying to build your Simple Android OCR project or rmtheis’s OCR Test, I haven’t been successful.
Thanks!
Yes–that should work. I build the library that way and share the tess-two folder in Shared Folders.
One thing to check would be to just try instantiating a TessBaseAPI object in onCreate() in a small test app to see if that works by itself first:
TessBaseAPI baseApi = new TessBaseAPI();
// then try baseApi.init() and check for messages in LogCat
baseApi.init("/mnt/sdcard", "eng", TessBaseAPI.OEM_TESSERACT_ONLY);
Thanks for your reply. I’ve tried your suggestion, but keep getting the native error, “Could not initialize Tesseract API with language=eng!” on init() when I try to build.
The error seems sort of vague and so far haven’t found any solutions online. Any ideas?
I think the problem was that I didn’t have the English language files in my tessdata folder.
ant release then following error:
/root/tess/tess-two/src/com/googlecode/leptonica/android/Jpeg10.IO:68 error: can not find symbol
symbol: variable FROYO
BUILD FAILED
/root/android-sdk-linux/tools/ant/build.xml:651 The following error occured while executing this line:
/root/android-sdk-linux/tools/ant/build.xml:672 Compiled failed; see the compiler error output for details
I am using android sdkr16, android ndk7 and ubuntu 11.04
Please help me …
Okey, I just successful build in jdk1.6. But Froyo can not resolved or is not field JpegIO.java, ReadFile.java, WriteFile.java.
I’m almost done configuring it on windows 7 with cygwin, however I encountered following error :
C:\Software\tess\android-ndk-r7c\tess\tess-two>android update project --path .
Error: The project either has no target set or the target is invalid.
Please provide a --target to the 'android.bat update' command.
How can I solve this? Here I am not sure what “project” refers to.
hi,
keep up the awesome work.I’ve developed an ocr system using opencv and tesseract. But i need to run it on atom kit which is loaded with windows 7(customized). Can you please give me your suggestions on this. I’m calling the tesseract from the command prompt. do i need to run tesseract on the atom kit as well? the kit has the vc++2010 redistributable.(app built in vc++ 2010).
Thanks
Hi, I followed your guide to run tess-two on Ubuntu 11.10. Everything went good until I had to update project with command :
android update project --path .
A following error occured :
Project either has no target set or the target is invalid. Provide option -t with update command.
So I provided the target with ID “1”, which has following description :
Android 4.0.3 , API level : 15, Revision : 3, ABIs : armeabi-v7a
and it updated correctly.
Then there was some error while importing the project in eclipse stating that something was wrong with “res” directory, but I did Fix Project Properties and it seems ok now. And now I am stuck at step when I should add libarary.. but I can’t. When I try to :
Right click your project name -> Properties -> Android -> Library -> Add, and choose tess-two, I can’t choose anything ( and there is nothing on the list, especially not “tess-two” ), OK button is disabled, and box named “Please select a library project” is also disabled.
Any ideas what to do know?
Thanks
Hi, I’m new to this so can you please tell me how to use ant release command. Im working on windows 7 PC. when I run it on command prompt it returns
C:\Users\raveen>ant release
'ant' is not recognized as an internal or external command, operable program or batch file."
solved it, my bad I forgot to set environment variable, any way great article this is. 🙂
What is and how to set DATA_PATH, lang in below line?
baseApi.init(DATA_PATH, lang); baseApi.setImage(bitmap);
Same problem as koleS’. I am stuck at step when I should add libarary, but I can’t. When I try to:
Right click your project name -> Properties -> Android -> Library -> Add, and choose tess-two, I can’t choose anything (and there is nothing on the list, especially not “tess-two” ), OK button is disabled, and box named “Please select a library project” is also disabled.
Any ideas what to do know?
Actually I did a silly thing, because I wanted to add the library to… itself!
When you set your library properly you then have to add it in another project, in which you want to use the library. Then options to add it will be enabled.
Thanks for this great tutorial it helped me greatly but can you be more specific about how to change the language of data using the training data in tesseract site.
By the way I did the steps in the tutorials and it works.
1. Download a new language data file and extract it to your data directory
2. Change the string parameter to baseApi.init to match the new language’s three-letter code
Do you need to do any “training” of the OCR engine? I’m trying to use this to read car registration plates, road signs etc, however rather than performing OCR on the given image, it attempts to “draw” it using characters, giving something that resembles ASCII art..
Any suggestions? Thanks
Try the HydrogenTextDetector class in the eyes-two project.
Training will help detect a particular font consistently, so I expect it would help for registration plates and road signs since they should always use the same fonts. But I don’t expect training to help find the text areas within a large image.
If you know you can expect your text and background colors to be consistent every time, you could try running custom thresholding based on that to pass a high-contrast image to the OCR engine.
I’m getting incredibly varied results, if I try the following image :
http://carandvannews.co.uk/wp-content/uploads/2012/04/12Plate.jpg
Then I get “YTI2 ULR” which is close enough.
However, if I try :
http://www.v1seo.co.uk/v1seo-polo.jpg
Then I get about 5 lines of nonsense, just special characters or random gibberish.
I’ve followed all the resources I can find, and have looked at your Github project, but I’m still not able to get any reliable recognition working across various images.
Is there some way of setting regions, so when trying to OCR a number plate it would look for a yellow/white rectangle and only OCR on that particular area? That would help improve results, but I can’t find any good examples.
Thanks
Not with Tesseract, but OpenCV or Leptonica may be useful.
Take a look at these:
http://stackoverflow.com/questions/4727119/continued-vehicle-license-plate-detection
http://stackoverflow.com/questions/4777677/license-plate-recognition-determining-color-range-for-pixel-comparison
http://stackoverflow.com/questions/4707607/what-are-good-algorithms-for-vehicle-license-plate-detection
Hi rmtheis and gautam,
I think i have compiled the entire code, i am able to run the app in emulator but as soon as i capture an image, the application crashes .
According to logcat and my understanding, the application is not able to get TessBaseAPI. It throws an java.lang.exceptionInInitializerError.
E/AndroidRuntime(547): Caused by: java.lang.UnsatisfiedLinkError: Couldn't load lept: findLibrary returned null
Please help. Thanks.
Did you add tess-two as a library project?
I’m facing the same error as sacsha, I already added the tess-two as library project, but still get that error, and I also try it to OCRTest sample project and it give the same error. I’ve try to run it in windows and mac and the error still the same, please help
Hey Gautam, I get this error when typing
android update project --path in command: "Error: Missing argument for flag --path"
. What’s wrong?You forgot the ‘.’ to indicate the current directory.
Hey Gautam, your tutorial is awesome and it runs perfectly for me.
But the output results are like 50%. 🙁
Can you please tell me other ORC libraries other than Tesseract to get text from images?
You might want to use the Google Docs API for OCR. Never tried implementing it myself though, but it does produce better results. 🙂
Thanks!
You can improve results dramatically by allowing the user to crop the image (if that fits with your model), also, try adding white/blacklisted characters.
What do mean by white/blacklisted characters James Elsey?
One thing more, every time when I install .apk file on a new android phone, I have used to place eng.traindata separately after installation. Is it possible that my akp file includes eng.traindata and when I install my apk it will automatically copy this file to a separate folder? Any helpful link?
Muhammad you need to copy it from the assets directory and onto your SD card when you load the app. That way, when a user installs the app and opens for the first time, the traineddata file gets deployed onto the SD card ready for use.
Black/whist listed characters allows you to ignore particular characters and look for others
Hi guys, I try to configure it on windows 7 using Cygwin, I can build with ndk-built but I have this error
-bash: android : commande introuvable when I try android update project --path
Despite I set environment variables for the sdk, the error persists.
I need help please. Thanks in advance!
You have to add the SDK’s tools/ and platform-tools/ directories to your PATH.
Could not find tess-two.apk! Could you please help me with this?
You can get it from bin folder under tess-two folder – https://github.com/rmtheis/tess-two
I have succesfully build the tess-two library on my ubuntu 12.04. But I still get force close error from my app. Do I have to use the leptonica processing library for rotating etc? Could you send me the full code that running your site? Thanks for your help!
What error do you see when you look at LogCat?
I am using your simple android app, I want to modify the program so that an image should be stored in gallery of a mobile – just taking the image and getting the text from the image. Please reply as soon as possible. Thanks!
Is it okay if I build the source code you provided on a windows laptop or for that too I need to do it on a linux machine?
The latest version should work on Windows.
Okay one more question – I downloaded the source code of ur simple OCR app. If i am directly using your code then I dont need to do that ndk-build and all those steps right? Sorry but i am new to this… would be glad if you could reply asap as this will be my project. Thanks. 🙂
You still have to do the ndk-build.
When I try to import the project, it is showing some problems –
"FROYO cannot be resolved or is not a field ReadFile.java /tess-two/src/com/googlecode/leptonica/android line 47 Java Problem"
also "ERROR: resource directory '/home/akhila/Desktop/android downloads/rmtheis-tess-two-071820a/tess-two/res' does not exist"
I have imported only the tess-two project.
Have you installed the Android SDK?
Hi there Gautam! I’m student from Taiwan. First thank you for writing this page.
I have one question about initializing Tesseract OCR engine for Chinese (traditional). I can run this app on my device with English but chinese is not working without fail. “Please Wait initializing Tesseract OCR engine for Chinese (traditional)” then close.
Could you tell me how to fix this? Thanks!
I have successfully made it work with Hindi, don’t know what could be the error for you.
It could be a RAM problem, because the Chinese model is bigger. Does it work on the emulator?
Hi Gautam,
I’m trying to build Hindi OCR android app.
You said you made it work with Hindi. Do we need to use .cube files also for Hindi language ? If yes, then how are we making use of .cube files and where to put these files ?
Thanks in advance
I am beginner for android platform. Please Can you explain me what is the technology you have used for image processing when using OCR. I mean histogram analyse, edge detection like that. Or please just give me road map how this is working. Have you used the match lab for this implementation?
You can Google on how does Tesseract work, there are some presentations and PDFs which will help you learn on the how-to. 🙂
Hi Gautam,
I am working on tesseract on linux. Tesseract doesn’t provide any support for OCRing image.
Can you please tell me how this support can be provided in tesseract? Do we need to write any new API or is there any existing API in tesseract code which can be modified to support image?
Do you know any API which was implemented for other OCR technique for images and the same can be included into tesseract?
Thanks, Anoop.
Hi Gautam, you are doing a mind blowing job! Am a newbie working on OCR right now.
I have built ndk in windows itself generated some 250 mb and .so files ina armeabi, armeabi-v7a and x86 folders and respective liblept and libtess.so. But I get ExceptionInInitializer error –
"06-03 12:22:56.745: E/AndroidRuntime(15568): Caused by: java.lang.UnsatisfiedLinkError: Library lept not found"
Can you please help me out?
You’re getting that error because the libraries are not available when your application runs.
Make sure you have them imported as library projects in your IDE and are available at application runtime (tess-two that is)
I am getting following error when app is running.
Ljava/lang/UnsatisfiedLinkError; thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI
It is getting in this line
baseApi = new TessBaseAPI();
It looks like unable to initialize TessBaseAPI.
What can I do for this? Is there any special OCR configuration ?
Hey Dharshana,
Is there anything more printed out in your logcat?
Make sure that you’re IDE is placing tess-two as a library project, and that it is actually getting deployed to the device.
Also make sure that the traineddata files are there, and are not secretly there but with 0 bytes (that caught me out).
Since you can’t even “new up” the TessBaseAPI, I’d wonder if its an issue with the tess-two library dependency not being available at runtime..
Hi james, I have the same problem, I placed tess-two as a library project and added it to my ocr project but I still have this error:
06-08 09:31:15.450: D/asset(252): Data exceeds UNCOMPRESS_DATA_MAX (1926792 vs 1048576)
06-08 09:31:15.450: E/SimpleAndroidOCR.java(252): Was unable to copy fra traineddata java.io.IOException
06-08 09:32:41.850: V/SimpleAndroidOCR.java(252): Starting Camera app
06-08 09:32:44.910: W/IInputConnectionWrapper(252): showStatusIcon on inactive InputConnection
06-08 09:32:52.302: D/dalvikvm(252): GC freed 1138 objects / 77784 bytes in 222ms
06-08 09:32:53.070: I/SimpleAndroidOCR.java(252): resultCode: -1
06-08 09:32:53.390: V/SimpleAndroidOCR.java(252): creation of the bitmap
06-08 09:32:53.450: V/SimpleAndroidOCR.java(252): Orient: 0
06-08 09:32:53.460: V/SimpleAndroidOCR.java(252): Rotation: 0
06-08 09:32:53.460: V/SimpleAndroidOCR.java(252): could correct orientation
06-08 09:32:53.470: V/SimpleAndroidOCR.java(252): could correct orientation
06-08 09:32:53.470: V/SimpleAndroidOCR.java(252): Before baseApi
06-08 09:32:53.480: D/dalvikvm(252): Trying to load lib /data/data/com.datumdroid.android.ocr.simple/lib/liblept.so 0x44e8cb20
06-08 09:32:53.830: I/dalvikvm(252): Unable to dlopen(/data/data/com.datumdroid.android.ocr.simple/lib/liblept.so): Cannot load library: link_image[1721]: 30 could not load needed library 'libjpeg.so' for 'liblept.so' (load_library[1051]: Library 'libjpeg.so' not found)
06-08 09:32:53.830: W/dalvikvm(252): Exception Ljava/lang/UnsatisfiedLinkError; thrown during Lcom/googlecode/tesseract/android/TessBaseAPI;.
06-08 09:32:53.840: D/AndroidRuntime(252): Shutting down VM
06-08 09:32:53.840: W/dalvikvm(252): threadid=3: thread exiting with uncaught exception (group=0x4001b188)
06-08 09:32:53.850: E/AndroidRuntime(252): Uncaught handler: thread main exiting due to uncaught exception
06-08 09:32:54.100: E/AndroidRuntime(252): java.lang.ExceptionInInitializerError
06-08 09:32:54.100: E/AndroidRuntime(252): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onPhotoTaken(SimpleAndroidOCRActivity.java:247)
06-08 09:32:54.100: E/AndroidRuntime(252): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onActivityResult(SimpleAndroidOCRActivity.java:164)
06-08 09:32:54.100: E/AndroidRuntime(252): at android.app.Activity.dispatchActivityResult(Activity.java:3828)
...
06-08 09:32:54.100: E/AndroidRuntime(252): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:47)
06-08 09:32:54.100: E/AndroidRuntime(252): ... 15 more
06-08 09:32:54.140: I/dalvikvm(252): threadid=7: reacting to signal 3
06-08 09:32:54.444: I/dalvikvm(252): Wrote stack trace to '/data/anr/traces.txt'
06-08 09:32:57.100: I/Process(252): Sending signal. PID: 252 SIG: 9
I need any help. Thanks in advance.
Ouch, you’re getting all sorts of horrible errors there!
Firstly you got an IOException trying to copy the traindeddata file over, is that the right filename? Do you have external storage write permissions? As a dirty hack, I’d copy it over manually now via DDMS file explorer, and come back and fix that later, its not ideal, but will get you progressing.
Next it complains about *.so files being missing, and subsequent errors are probably stemming from this. Looks to me like the library project is not there, as your app can’t find files from it.
If you’re a fan of Maven, I’d seriously recommend trying to use that as its library management is pretty good, I wrote a quick tutorial here:
http://www.jameselsey.co.uk/blogs/techblog/tesseract-ocr-on-android-is-easier-if-you-maven-ise-it-works-on-windows-too/
Hi James thanks for reply, but there is no other solution to make it working without maven, I try with android 2.2 but I have the same problem. I need help please. Thanks in advance
I am using windows. I have compiled and build project successfully. But it is not working. When running and it says
Ljava/lang/UnsatisfiedLinkError; thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI.
I did testing after commented TessBaseAPI initializing line then it is working and showing UI with square. But it was failed when getting the image snap from the camera. Then it says no OCR plugging.
I am using windows. Still I can’t understand what the usage of ndk-build
is. What is usage of it? Do I need to build NDK using cygwin. I have configured test-two project as well.
Is it possible run this in windows with Cygwin or do I need to use Linux?
Please help me because this is very critical for me now.
I t would be highly appreciated if you can help me to run this project.
Hi dharshana, yes it is possible to run this on windows. I have successfully build tess-two on windows with cygwin. Then I use android 2.3 emulator – I don’t have errors but I have a bad result.
I have used NDK and tried to build test_two project from Cygwin. While running NDK build command I have got following error. Please help me.
Compile thumb : lept <= pix4.c
...
Compile++ thumb : lept <= jni.cpp
Prebuilt : libgnustl_static.a <= /sources/cxx-stl/gnu-libstdc++/libs/ armeabi/
SharedLibrary : liblept.so
F:/MSC_project/android-ndk-r8/toolchains/arm-linux-androideabi-4.4.3/prebuilt/wi ndows/bin/../lib/gcc/arm-linux-androideabi/4.4.3/../../../../arm-linux-androidea bi/bin/ld.exe: ./obj/local/armeabi/libgnustl_static.a: No such file: Permission denied
collect2: ld returned 1 exit status
/cygdrive/f/MSC_project/android-ndk-r8/build/core/build-binary.mk:369: recipe fo r target `obj/local/armeabi/liblept.so' failed
make: *** [obj/local/armeabi/liblept.so] Error 1
I am glad to say, it was possible to build test_two project from NDK build without errors. I will do rest in next day and update you. The issue was my laptop folder permission issue. But I had to work with this issue until yesterday late night around 3 am. (:
I have the same problem, how can I fix it?
I need know how to setup base language and target(translating) language. I mean where to add Tesseract libs. I need to setup base language as Sinhala and translating (target) language is English. Is it possible? Then how can I do that? Please help me.
hey Dharshana,
You have 2 very distinct requirements there, I would seriously recommend splitting that work out and trying to do one thing at a time.
First off, you need to get tesseract working with your target language. Once you can get that to log out the text, then you can begin to look at the translation (there are google APIs for that, dead easy to use)
What is Sinhala? You can find the traineddata files for all the supported languages on the tesseract site here :
http://code.google.com/p/tesseract-ocr/downloads/list
Hi James Elsey
Yes I have 2 requirements for this.
I need to read Sinhala notice board or newspaper text from mobile phone and translate to English.
Sinhala is the language using in Sri Lanka.
My objective is Sinhala text translates to English.
Please advise me where I have to setup application base language as Sinhala.
Regards
Dharshana
Setup of base language is done using init().
At the link James referenced, Sinhala is not on the list of languages supported, so you would have to create the appropriate data file:
http://code.google.com/p/tesseract-ocr/wiki/TrainingTesseract3
$ ndk-build
Install : libjpeg.so => libs/armeabi/libjpeg.so
SharedLibrary : liblept.so
E:/AndroidStuff/android-ndk-r8/toolchains/arm-linux-androideabi-4.4.3/prebuilt/windows/bin/../lib/gcc/arm-linux-androideabi/4.4.3/../../../../arm-linux-androideabi/bin/ld.exe: ./obj/local/armeabi/libgnustl_static.a: No such file: Permission denied
collect2: ld returned 1 exit status
/cygdrive/e/AndroidStuff/android-ndk-r8/build/core/build-binary.mk:369: recipe for target `obj/local/armeabi/liblept.so' failed
make: *** [obj/local/armeabi/liblept.so] Error 1
The latest tess-two does not use LibJpeg. Use the latest code.
Thanks for replying! I have infact used the latest code. Can you please try to compile it on a Windows machine and see if it works?
It works fine on Windows 7 but I’m not sure about Cygwin. Do “ndk-build clean” and try again without Cygwin. If you see references to “libjpeg.so” then you’re using old code.
It is working fine in windows 7. I have done everything correctly, Cygwin build also worked.
Hi, very nice Tut,
I am on a Mac and have run the ndk-build ok.
but
android update project --path .
Fails with
Error: The project either has no target set or the target is invalid.
Please provide a --target to the 'android update' command.
what is this trying to do and any tips for fixing it
Thanks
android list targets,
comes back empty ?
I had a look at project.properties
cat project.properties
android.library=true
# Project target.
target=android-15
and so I try
android update project --path . --target android-15
Error: Target id ‘android-15’ is not valid. Use ‘android list targets’ to get the target ids.
no luck cus i dont know what i am doing
If you have problem with command:
Put in terminal to check ID of your target:
Then put:
Hi Gautam
I did try out with your points to creating tess-two project. I did even import to Eclipse, but when I add this project as library and when I try to run using android emulator I get exception as in emulator as
W/PackageManager(58): Failed to cache package shared libs
W/PackageManager(58): java.io.IOException: Couldn't create cached binary /data/data/com.uni.demo/lib/libtess.so in /data/data/com.uni.demo/lib
W/PackageManager(58): at com.android.server.PackageManagerService.cacheNativeBinaryLI(PackageManagerService.java:3771)
...
W/PackageManager(58): Package couldn't be installed in /data/app/com.uni.demo-1.apk
Looking forward to your reply.
thanks.
Hi I did solve the exception that was coming in the project and I totally built it on windows. One more question how to set an area(which is expandable and reduce able) to capture the text.
Looking forward to your reply.
thanks.
You’re best bet is to allow the user to crop the image, at least that way you can be certain you’ll only OCR the targetted area and not pick up other things that you’re not interested in.
Hi, you can take a look at this open source app by rmtheis which would result in something like the picture shown in this blog post – https://github.com/rmtheis/android-ocr
Hi
Please can u help me to find where I have to put “tesseract-ocr-3.01.osd.tar.gz” file in the “rmtheis-android-ocr-1e83e96” project. I need to use this application without downloading any data from the internet.
thanks
You can write some code to install it from assets/ or you can run without OSD using PSM_AUTO.
In addition to that I need to know where I have to put “tesseract-ocr-3.01.eng.tar.gz” file in the project.
In the assets folder.
I cant understand how to update Path variables as it does not expand in the troubleshooting section.can u put it in the comments plz
Thank you for the replay earlier about changing the language, I tried what you said but the program just shuts down without any warning. I’m trying to make the program read arabic but always the same thing. Here is my logcat if you can help I’d be grateful
06-16 15:41:09.001: I/DEBUG(31): beac8eb4 66206e69
06-16 15:41:10.171: D/Zygote(33): Process 696 terminated by signal (11)
06-16 15:41:10.171: I/ActivityManager(70): Process com.datumdroid.android.ocr.simple (pid 696) has died.
06-16 15:41:10.181: I/WindowManager(70): WIN DEATH: Window{409c3ec0 com.datumdroid.android.ocr.simple/com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity paused=false}
06-16 15:41:10.191: I/WindowManager(70): Setting rotation to 0, animFlags=1
06-16 15:41:10.201: I/ActivityManager(70): Config changed: { scale=1.0 imsi=310/260 loc=en_US touch=3 keys=2/1/2 nav=3/1 orien=1 layout=34 uiMode=17 seq=17}
06-16 15:41:10.981: W/IInputConnectionWrapper(452): showStatusIcon on inactive InputConnection
06-16 15:41:12.721: D/dalvikvm(70): GC_EXPLICIT freed 39K, 60% free 4181K/10375K, external 3125K/3903K, paused 75ms
Good Day Gautam!
I have successfully solved the error “./tess-two/res’ does not exist”. So I tried running the Simple OCR app you made as my guide in making my own. When I tried to run it as Android Application, a window will appear saying “Android library projects cannot be launched”
Is that okay? Will it be running successfully on a device?
I do not have any android phone with me at the moment that’s why I’m using the emulator.
You have check Simple OCR as a library.
Only Tess-two must be a library, try to check that and it might work.
hey how did u solve that error??
Please help me
I already have the tess-two ‘s jar and so and added them into my project.
when the AP runs to the code “baseApi.setImage(bitmap);” I got the errors as below:
W/dalvikvm(20016): No implementation found for native Lcom/googlecode/tesseract/android/TessBaseAPI;.nativeSetImageBitmap (Landroid/graphics/Bitmap;)V
06-28 05:57:03.080: W/dalvikvm(20016): threadid=1: thread exiting with uncaught exception (group=0x409c01f8)
06-28 05:57:03.206: E/AndroidRuntime(20016): FATAL EXCEPTION: main
06-28 05:57:03.206: E/AndroidRuntime(20016): java.lang.UnsatisfiedLinkError: nativeSetImageBitmap
06-28 05:57:03.206: E/AndroidRuntime(20016): at com.googlecode.tesseract.android.TessBaseAPI.nativeSetImageBitmap(Native Method)
06-28 05:57:03.206: E/AndroidRuntime(20016): at com.googlecode.tesseract.android.TessBaseAPI.setImage(TessBaseAPI.java:246)
06-28 05:57:03.206: E/AndroidRuntime(20016): at com.besta.app.camdict.CamDictActivity.ocr(CamDictActivity.java:168)
06-28 05:57:03.206: E/AndroidRuntime(20016): at com.besta.app.camdict.CamDictActivity$ButtonClickHandler2.onClick(CamDictActivity.java:76)
06-28 05:57:03.206: E/AndroidRuntime(20016): at android.view.View.performClick(View.java:3511)
...
06-28 05:57:03.206: E/AndroidRuntime(20016): at dalvik.system.NativeStart.main(Native Method)
What jar? Follow the instructions: The project is added as a library project, not as a jar.
It’s tess-two.tesseract3.01-leptonica1.68-LibJPEG6b.jar
The so files and jar are compiled by someone else.
The project “tesseract-android-tools” has been added as a library.And the tessdata has coped into SDcard.
Hi,
So i followed all the steps and even updated my PATH variables in my .bashrc file and extracted the tesseract and did ndk-build but i am getting stuck on the android update project –path step ..can anyone explain to me what am i exactly supposed to type here .. i did type android update project –path /home/mustafa/Downloads/tess/tess-two but it showed me an error which says Missing argument for flag
Thanks.
cd /home/mustafa/Downloads/tess/tess-two
android update project –path .
If this is unclear, check the instructions at https://github.com/rmtheis/tess-two
Please can anyone explain why following all file are using in the application? It would be nice if it can be explained file by file. Why it is using what will happen if I do not use it likewise.
eng.cube.bigrams
eng.cube.fold
eng.cube.lm
eng.cube.nn
eng.cube.params
eng.cube.size
eng.cube.word-freq
eng.tesseract_cube.nn
eng.traineddata
osd.traineddata
These are downloading when app is restarting at first time.
Hi rmtheis
In the file
OcrInitAsyncTask.java
there is an comment and the code
// Check assets for language data to install. If not present, download from Internet
try {
Log.d(TAG, "Checking for language data (" + destinationFilenameBase
+ ".zip) in application assets...");
// Check for a file like "eng.traineddata.zip" or "tesseract-ocr-3.01.eng.tar.zip"
installSuccess = installFromAssets(destinationFilenameBase + ".zip", tessdataDir,
downloadFile);
I tried with adding files in assets folder rather than to download from the internet.
But this is not working. Tried with debugging and gives me back as file not found. Any code help on this would be useful.thanks.
Post your question to StackOverflow and include your LogCat output along with the Java code you’ve posted here.
Just write the file transfer method and try transfer required files from the assets folder to application. I also did that. You can see the sample code in Simple-Android-OCR project.
The error i had while including the Simple-Android-OCR
http://stackoverflow.com/questions/10560144/force-close-after-run-android-simple-ocr-aplication
Even though i have already included in manifest.xml
The log cat i have got:
07-13 15:55:30.424: E/AndroidRuntime(26781): java.lang.ExceptionInInitializerError
07-13 15:55:30.424: E/AndroidRuntime(26781): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onPhotoTaken(SimpleAndroidOCRActivity.java:217)
07-13 15:55:30.424: E/AndroidRuntime(26781): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onActivityResult(SimpleAndroidOCRActivity.java:140)
07-13 15:55:30.424: E/AndroidRuntime(26781): at android.app.Activity.dispatchActivityResult(Activity.java:3890)
...
07-13 15:55:30.424: E/AndroidRuntime(26781): at com.googlecode.tesseract.android.Te
I want to design an app as a project,so
I downloaded Simple-Android-Ocr project (thnx a lot for it Gautam)but now when i went to its Java Build Path->Android Dependencies->tess-two.jar.Here it shows the path of tess-two.jar as=E:\AndroidProject\tess-two\bin(missing)
plz could any one tell me how to solve this missing issue?
and
(there is no error marking seen for the project in the Project Explorer but a red exclamation mark,Why?)
You might want to try asking this question on StackOverflow.
Be sure to leave as many details as you can, including exactly how you built the library.
Just create a dummy folder like “bin” inside the test_two project and try.
Hi Rmtheis && Gautam
I have completely run the your simple and advance applications and it was successful. I am doing a project for my MSC which relates to this area. I will do some improvement with OCR. I will update u when it will success.
Thank you very much for your kind support and maintaining this blog.
Regards
Dharshana
Okay, this will blow your mind. I’ve achieved Object/Image Recognition, in an app. I won’t even begin to go into the code.. it was ridiculous. Basically I use algorithms I developed to scour the net and do many calculations:)
Check it out, particularly the video, it scared me when I first started debugging it!!
Computer Vision!!!! Finally! Android Eye is the first Object Recognition App. Take a picture of any object, and Android Eye will tell you what it is. Computer Vision. Image Recognition Technology (IRC). Take a picture of a car… Android Eye will tell you the make and model of the car. Take a picture of a foreign t-shirt label… Android Eye will tell you the brand, and where the shirt is from. Take a picture of a tree… a ball… a person… the results are endless. This is the Beta version, it works very well, particularly with vehicles, products, brands, and well-known “things”:) Software that does this is usually only available to government agencies and research facilities. I will eventually release the code “open source”, once it’s been on the market for a bit:)
Enjoy, and learn with it:) Ideal for visually impaired persons. Ideal for identifying vehicles or other manufactured items including computers, phones, or anything you would like a name for, a make or a model:)
https://play.google.com/store/apps/details?id=com.davecote.androideyes
http://www.youtube.com/watch?v=YEArrqAoL8A
Dave:)
Hi Gautam,
I got the demo working, all the way up to taking a picture. But when I try the OCR, the app closes without warning.
In the catlog, I see this:
07-31 23:35:49.745: I/DEBUG(1251): pid: 32060, tid: 32060 >>> com.example.ocrdemo <<<
07-31 23:35:49.745: I/DEBUG(1251): signal 11 (SIGSEGV), code 1 (SEGV_MAPERR), fault addr 00000000
07-31 23:35:49.745: I/DEBUG(1251): r0 0000009e r1 afd42428 r2 afd435d8 r3 00000000
…
This happens at or just before the line:
baseApi.init(DATA_PATH, lang);
Do you have any idea what is the matter here?
Thanks in advance,
Wim.
Hi Gautam i am trying to make an ocr based application in android using these instructions but getting error when trying to run this command “cd /tess-two ndk-build android update project –path . ant release
” . The error is “‘ndk-build’ is not recognized as an internal or external command,operable program or batch file” any help will be appreciated .thank you ..
For me libjpeg.so could not create. I’m following as per your instruction would you please help me.
Hi,
I am thinking to develop an open source mobile application to read license plates in order to retrieve the number with the cell phone. I mean, with the cell phone I take a picture of the car (front or back), and then the application performs an OCR analyzes in order to retrieve the matriculation number. Once I have the text in the application, I could realize other operations, such as report the license plate to a Police central via SMS.
I saw that your project performs OCR on Android, and this is a starting point for my idea, but probably this is the most important part because of the OCR algorithm. I would like to know if your project could really help me with the idea, and also, it would be very interesting to know your opinions.
Finally, taking what you say in the profile, are you interested in being part of this idea I am proposing?
Thanks, and good work.
Sorry, but I’m not available as I’m busy with other work. 🙂
You can read number plate or any other word in the picture which is captured from Android device using following URL.
http://rmtheis.wordpress.com/2011/08/06/using-tesseract-tools-for-android-to-create-a-basic-ocr-app/
I have run completely this code and now I can read any text from image from getting the Android camera. This can work even real time video as well without getting image.
Hello, I’m trying to build this library on Windows Vista. Reading these posts, I’m realizing that building tesseract will likely not be a possibility for me. Is there a place where I can download the already built library project for eclipse? I’m leaving for MIT in two days so I won’t be able to work on this anytime soon. Thanks in advance
I think it should work on Vista.
http://developer.android.com/tools/sdk/ndk/overview.html#reqs
For example I have an image in which has the number in it. Is it possible to read the number from the image? Even if the image is black/white or color image. Can you please suggest any ideas?
I have followed all the steps mentioned above and its not showing any errors. But at the runtime i am getting the ExceptionInInitializerError while initializing the TessBaseAPI object. Can you pls help me with that problm? Thank you.
Hi Gautam.
I tried your code for recognizing the text from the image the code and results are fine, but when I try with the image that have numbers, the app mistankenly gives the values wrong sometimes. Can you please suggest any solution?
Thanks Gautam. It’s good tutorial!
I’m compiling tesseract-android-tools. I found an error which I can’t fix. I posted it on http://stackoverflow.com/questions/12437533/error-in-compiling-tesseract
Please help me.
Hello Gautam. I’ve compiled the code completely on my Windows 7, there’s no problem, despite that I never get reasonable results from reading my pictures. Do you know some reasons for this?
I tried later to limit the photo size to less than 30kb and it began to recognize the words…
Heya i’m for the first time here. I found this board and I find It truly useful & it helped me out much. I hope to give something back and help others like you helped me.
Hey Gautam,
Seriously your post help me too much.
So thank you.
You are genius. 🙂
Gautam,
Thank you very much for this blog. I am just a beginner on Android development. I was able to set up the project in my Eclipse and able to run the simple android ocr app.
I had to provide the target number and also had to re-install the JDK and provide proper JAVA_HOME variable while doing step#2. Other than that the step step instruction was very helpful.
NB: I am using windows7 OS and it worked properly.
Way to go buddy!!
Santhosh
Thank you again!!
Hi Everybody
I run the ndk-build command and getting error
please give me response this
/cygdrive/d/android-ndk-r8b/ndk-build
Cygwin : Generating dependency file converter script
Compile thumb : lept <= open_memstream.c
Compile thumb : lept <= fopencookie.c
Compile thumb : lept <= fmemopen.c
Compile++ thumb : lept <= box.cpp
In file included from jni/com_googlecode_leptonica_android/box.cpp:17:0:
jni/com_googlecode_leptonica_android/common.h:22:24: fatal error: allheaders.h: No such file or directory
compilation terminated.
/cygdrive/d/android-ndk-r8b/build/core/build-binary.mk:255: recipe for target `obj/local/armeabi/objs/lept/box.o' failed
make: *** [obj/local/armeabi/objs/lept/box.o] Error 1
Hi Irfan; how did you manage to solve the allheaders no such file error? Having that here, using android studio.
Thanks Gautam, I am new to both Android and OCR processing, but your tutorial was significant great on my first move on them. Thanks a lot!
By the way I have done this tutorial by Eclipse on Window 7 with the help of cygwin and apache-ant-1.8.4.
Let’s work hard together for a bright future, all the best! 🙂
Hi Gautam,
I want to use leptonica tools in android for document image analysis . Any idea how to do that ?
Thanks in advance
Me too, especially for id cards. I’ve trained my own tesseract data, and seen a big improvement in recognition, but still no idea for how to specifically analyse the cards….
xinton, you mention you’ve trained your own tesseract data? Could you explain to me exactly how you train tesseract for android? As in what would I download, the procedure, then how do I get it back into android? I’ve looked at this http://code.google.com/p/tesseract-ocr/wiki/TrainingTesseract3 but couldn’t understand most of it.
just prepare a sample photo of your own font, open the command line, and follow the instructions on that site carefully. it’s no that difficult. you don’t need the understand the site completely, you will understand it gradually, don’t worry
Hi all,
I have followed this tutorial accurately. However, the line
TessBaseAPI baseAPI = new TessBaseAPI();
causes my Android app to shut down unexpectedly. Upon looking at logcat, it says that this caused because of missing Library lept. The exact line is:
10-16 16:24:16.406: E/AndroidRuntime(389): Caused by: java.lang.UnsatisfiedLinkError: Library lept not found
I have imported all the relevant libraries, including com.googlecode.tesseract.android.*
com.googlecode.leptonica.android.*
Any ideas what could be the reason and how to solve it?
Thanks!
Maybe you can go to the tess-two library, and see if you can find the file liblept.so in there? If not, you need to ndk-build your jni source
hi i am not able to run the code on windows xp i have downlaod cygwin and ndk 1.8 i am getting this error ExceptionInInitialiazer
please help me.
Hi,I have written an android application using tesseract-ocr after reading your post.I’ve to finished it but I need the user to edit the captured bitmap image for good result.Here is a question about it ,please help!This is the last step to publish my application.Good luck! http://stackoverflow.com/q/12931603/1665807
Thank You for such a wonderful tutorial. This works on Windows. No need of Cygwin. I used GitBash. But I wanted to ask that how do we increase accuracy?? I mean this app when run on mobile gives correct text once in 10 times.
Hi. When I run the Robert Theis app. (Intermediate+). I get “Could not initialize the camera. Please restart the device’. What could be the error? Any help would be appreciated 🙂
Somehow app worked :). Thanks to gautam and all those who helped me 🙂
Hi! I found a bug on running my app using Tesseract-ocr. You can see here.http://code.google.com/p/tesseract-android-tools/issues/detail?id=40&can=1
Hello. I’ve successfully compiled and run TessTwo in Eclipse Indigo on Windows for my Android app. It works ok with a short line of text (just one misread of a 2 as a Z). But if I increase the length of the text, at some point GetUTF8Text will crash my app. So, I’m trying to debug the jni native C code. I’ve compiled the C source (a long process), but still can’t get the debugger to stop ignoring my breakpoints in the C code. Can anyone offer any ideas? Thanks!
At what point does it crash? Large images can crash the emulator if you don’t have enough RAM defined.
What’s the message you see in LogCat?
Thanks for responding. I haven’t been able to debug any deeper than the nativeGetUTF8Text() call in the Tessbaseapi.class file. I can’t get a responsive breakpoint in the Tessbaseapi.java file at all. If I F5 on the GetUTFText call while debugging in Eclipse, the debugger will report only:
TessBaseAPI.nativeGetUTF8Text() line: not available [native method]
Still trying to get native debugging working properly in Eclipse. Haven’t even touched tessbaseapi.cpp yet.
In LogCat, using a ‘TessBaseAPI.java’ Log Tag filter (do you suggest any other?), I see only the following series of (probably irrelevant) messages:
11-04 23:42:47.505: D/TessBaseAPI.java(4075): finalize(): NOT calling nativeFinalize() due to premature garbage collection
11-04 23:42:47.505: D/TessBaseAPI.java(4075): finalize(): calling super.finalize()
Well, trying to debug the JNI code sounds like an exercise in frustration. Does it give a SIGSEGV message when it crashes?
I don’t see this kind of crash in my android-ocr project.
Yes, it’s frustrating. Did/do you ever have to debug your jni code? If so, how? No, I haven’t seen that SIGSEV message. In Log Cat? If so, what filter so as to isolate it from the other messages pouring into Log Cat?
Hi,
Great guide! finally got it working! 🙂
The only problem is that I get very bad results. The engine does not recognize most images I put threw it. I tried taking the pictures with the phone camera, and I tried downloading images from the web.
On both cases I get very very bad results. I even used the android ocr application and the simple application. Got bad results from it too.
Any ideas on how I can get better results??
Thanks!!
read through this blog and you’ll find:
1.cut the image util it contains only the text zone
2.augment the quality of your image with thresholding and many others means
Hello , I am a student in Jordan University Of Science And Technology (JUST) . Thanks Guatum for your posts , but I have a problem when running sentence translation (ocr) application in my laptop which work on Ubuntu 11.04 , when I download the project it is run but the emulator give me message say that (the application has been stopped unfortunately ) and I don not know why . Please help me because the due date of this project is next week.
master Gautam. can you make a tutorial on how to put movable borders on capturing words just like on the video
You can find the source code for the project to do that here. 🙂
@xinton
read through this blog and you’ll find:
1.cut the image util it contains only the text zone
2.augment the quality of your image with thresholding and many others means
Please elaborates these two steps clearly by code as well as possible…
Thank You
Is the ndk library necessary to import when using ocr translation?
Thank you Gautam. Works perfectly for me.
Can I use any library for translation other than google API? Because when I import the application the linked to google API library translation give an error.
Hi Gautam iam student in faculty of computer and informatics and I want to use tesseract with android these steps you have written is good but there is exception thrown is classDefNotFoundException event the tessBaseApi.class?
Hello Gautam hope you are doing well. Actually i want develop an application using OCR and on searching i found your blog. I have downloaded your tess-two-master tree from github and trying to run it. I am running it on Windows XP that’s why I have installed Cygwin and NDK and have set their path into the PATH variable. But on giving the ndk-build command from command prompt I’m getting this error:-
/usr/bin/sh: -c: line 1: syntax error: unexpected end of file make: *** [obj/local/armeabi/objs/lept/src/src/adaptmap.o] Error 1
I have searched this file in the tess-two-master folder but not found any adaptmap.o file.
Please help me on this.
Thank you in advance.
The Android NDK is compatible with Windows XP, so I suggest trying the build directly under Windows and not using Cygwin.
It work fine on windows 7, CYGWIN isn’t needed. I just had a little problem with my antivirus(COMODO) and I had to deactivate Defense+.
Can you tell me how you got working windows?
Mine built ok but when i try to do a ocr capture the app forces close
Hi Guys, Hi Gautam.
Good day.
We just started our thesis and it’s a Signage & word Translator using OCR for Android.
We already put the codes in Eclipse, compiled our scripts using leptonica and cygwin. Read all the steps, but we’re still getting errors.
We can’t compile them properly.
Listed in here are the errors we got:
1. [2012-11-28 02:53:20 – tess-two] Android requires compiler compliance level 5.0 or 6.0. Found ‘1.4’ instead. Please use Android Tools > Fix Project Properties.
2. [2012-11-28 02:53:21 – tess-two] Android requires compiler compliance level 5.0 or 6.0. Found ‘1.4’ instead. Please use Android Tools > Fix Project Properties.
3. [2012-11-28 02:53:49 – tess-two] ERROR: resource directory ‘C:Usersgatewayandroid-sdkstess-twores’ does not exist
4. [2012-11-28 02:58:06 – tess-two] ERROR: resource directory ‘C:Usersgatewayandroid-sdkstess-twores’ does not exist
Please.. Please..
Can you help us? 🙁
Thank you..
Right-click on the project name in Eclipse and choose Android Tools->Fix Project Properties.
Then do Project->Clean.
Thanks Gautam and Rmtheies for these great tutorial , i really appreciate your help god bless you.
I did all the step then when run my application and capture an image it does but when i press ok after an image capture it fails .when i look at logcat shows:
logcat:
11-27 23:39:43.527: I/SimpleAndroidOCR.java(1317): resultCode: -1
11-27 23:39:43.647: D/dalvikvm(1317): GC freed 1422 objects / 92160 bytes in 96ms
11-27 23:39:43.927: V/SimpleAndroidOCR.java(1317): Orient: 0
11-27 23:39:43.927: V/SimpleAndroidOCR.java(1317): Rotation: 0
11-27 23:39:43.957: D/dalvikvm(1317): GC freed 169 objects / 39536 bytes in 33ms
11-27 23:39:43.977: V/SimpleAndroidOCR.java(1317): Before baseApi
11-27 23:39:43.977: D/dalvikvm(1317): Trying to load lib /data/data/sms.recieving/lib/liblept.so 0x44717aa0
11-27 23:39:43.977: I/dalvikvm(1317): Unable to dlopen(/data/data/sms.recieving/lib/liblept.so): Cannot load library: link_image[1721]: 51 could not load needed library 'libjnigraphics.so' for 'liblept.so' (load_library[1051]: Library 'libjnigraphics.so' not found)
11-27 23:39:43.977: W/dalvikvm(1317): Exception Ljava/lang/UnsatisfiedLinkError; thrown during Lcom/googlecode/tesseract/android/TessBaseAPI;.
11-27 23:39:43.987: D/AndroidRuntime(1317): Shutting down VM
11-27 23:39:43.987: W/dalvikvm(1317): threadid=3: thread exiting with uncaught exception (group=0x4001b180)
11-27 23:39:43.987: E/AndroidRuntime(1317): Uncaught handler: thread main exiting due to uncaught exception
11-27 23:39:43.997: E/AndroidRuntime(1317): java.lang.ExceptionInInitializerError
11-27 23:39:43.997: E/AndroidRuntime(1317): at sms.recieving.ocrActivity.onPhotoTaken(ocrActivity.java:211)
11-27 23:39:43.997: E/AndroidRuntime(1317): at sms.recieving.ocrActivity.onActivityResult(ocrActivity.java:133)
...
11-27 23:39:43.997: E/AndroidRuntime(1317): at java.lang.System.loadLibrary(System.java:557)
11-27 23:39:43.997: E/AndroidRuntime(1317): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:47)
11-27 23:39:43.997: E/AndroidRuntime(1317): ... 15 more
11-27 23:39:43.997: I/dalvikvm(1317): threadid=7: reacting to signal 3
11-27 23:39:44.007: I/dalvikvm(1317): Wrote stack trace to '/data/anr/traces.txt'
Not sure. I would try updating your Android SDK and rebuilding tess-two.
Hi you have a great tutorial here but I really want to know does this also work for numbers?
Hey Gautam!
I am trying to run the initial set of commands for tess-two..on windows7 command Prompt.
For ndk-build am getting the following error:
Android NDK: Could not find application project directory !
Android NDK: Please define the NDK_PROJECT_PATH variable to point to it.
E:\android-ndk\android-ndk-r8c\build/core/build-local.mk:130: *** Android NDK: A
borting . Stop.
To Jack,
Yeah, it works fine for numbers.
To Meghna,
I’m not sure that it will work or not. I worked in Linux. May i know where the tess-two is kept?
you need to change directory first.(wrinting linux command, varies / or \ with this, may be)
cd /home/*your account name*/AndroidApc/tess-two/
/home/*your account name*/Documents/Android_NDK/ndk-build
it may help.
Thank you 🙂 That really helped.
Good Day Gautam and rmtheis
Can you help me solve this problem?
BUILD FAILED
/home/gateway/Downloads/tess-two-master/tess-two/build.xml:46: sdk.dir is missing. Make sure to generate local.properties using 'android update project' or to inject it through an env var
Thank you.
How did you solve the problem?
Could someone please tell me what should I update the path variable as – for ant release command.
I have downloaded ant, the path for that folder is E:\ant\apache-ant-1.8.4
To Meghana,
use sdk then update project then release ant.
Why is it the SimpleOcr cannot be installed in my android phone? I tried to export it with unsigned application project to build apk.
Did you change the setting on your phone to allow installation of non-Market apps?
How can we create a similar application for recognising other languages in android?
How can I specify the language to be used by tesseract?
As a three-letter language code in init(). It should correspond to the traineddata file.
well , i have changed the trained data from eng(english) to hin (hindi) and in the code changed the letters accordingly.It previously performed well for english characters, but didnt turn up with results with hindi letters.
Does
String recognizedText = baseApi.getUTF8Text();
have any issues with this??
thanks rmt, it worked by correcting the data file path correctly
🙂
how done it sam,i have same problem
@rmtheis
When I’m trying to export your ocr master why is this happening?
proguard returned with error code 1. see console
On the other hand @Gautam simpleOCR I can export it without any error considering the fact that I’m using both of your work on the same ADT?
Please help me. Thank you so much!
You need to use the Proguard config that’s in the project. What does the console say?
Thank you for the concern @rmtheis
The console says:
[2013-01-03 08:55:03 - OCRTest] Unexpected error while evaluating instruction:
[2013-01-03 08:55:03 - OCRTest] Class = [android/support/v4/view/AccessibilityDelegateCompat$AccessibilityDelegateJellyBeanImpl]
[2013-01-03 08:55:03 - OCRTest] Method = [newAccessiblityDelegateBridge(Landroid/support/v4/view/AccessibilityDelegateCompat;)Ljava/lang/Object;]
[2013-01-03 08:55:03 - OCRTest] Instruction = [18] areturn
[2013-01-03 08:55:03 - OCRTest] Exception = [java.lang.IllegalArgumentException] (Can't find any super classes of [android/support/v4/view/AccessibilityDelegateCompatIcs$1] (not even immediate super class [android/view/View$AccessibilityDelegate]))
[2013-01-03 08:55:03 - OCRTest] Unexpected error while performing partial evaluation:
[2013-01-03 08:55:03 - OCRTest] Class = [android/support/v4/view/AccessibilityDelegateCompat$AccessibilityDelegateJellyBeanImpl]
[2013-01-03 08:55:03 - OCRTest] Method = [newAccessiblityDelegateBridge(Landroid/support/v4/view/AccessibilityDelegateCompat;)Ljava/lang/Object;]
[2013-01-03 08:55:03 - OCRTest] Exception = [java.lang.IllegalArgumentException] (Can't find any super classes of [android/support/v4/view/AccessibilityDelegateCompatIcs$1] (not even immediate super class [android/view/View$AccessibilityDelegate]))
[2013-01-03 08:55:03 - OCRTest] java.lang.IllegalArgumentException: Can't find any super classes of [android/support/v4/view/AccessibilityDelegateCompatIcs$1] (not even immediate super class [android/view/View$AccessibilityDelegate])
[2013-01-03 08:55:03 - OCRTest] at proguard.evaluation.value.ReferenceValue.generalize(ReferenceValue.java:287)
[...]
[2013-01-03 08:55:03 - OCRTest] at proguard.ProGuard.main(ProGuard.java:492)
What happens if you set Project Properties->Android to 4.1?
I haven’t tried to set its properties to android 4.1 so far I’m using 3.1?
What should I write instead of DATA_PATH in this line baseApi.init(DATA_PATH, “eng”) ?
If your
eng.traineddata
is at/mnt/sdcard/tesseract/tessdata/eng.traineddata
then you should write
baseApi.init("/mnt/sdcard/tesseract/", "eng");
what is the eng.traineddata? how can I find its path?
Same doubt.
Do you solve it ?
Thanks
check the language packs available as downloads from the tesseract-ocr site. Download, unzip, copy to the tessdata directory.
https://code.google.com/p/tesseract-ocr/downloads/list
I am stuck at the command:
android update project –path .
So I set the PATH to:
C:\Users\SilverSand85X\Desktop\android-ndk-r8d;
C:\adt-bundle-windows\sdk\tools;
C:\Users\SilverSand85X\Desktop\OCR\tess-two-master;
C:\adt-bundle-windows\sdk\platform-tools
Am I doing it wrong?
Also does the OCR/program works on Windows 8?
The ndk-build works on Windows 8.
You don’t have to change your PATH, just type the command as is.
I have followed all of the instructions that have been given gautam, but I still get erorr when the running on my phone. And this is my Logcat:
01-27 19:08:36.182: E/AndroidRuntime(12957): FATAL EXCEPTION: main
01-27 19:08:36.182: E/AndroidRuntime(12957): java.lang.ExceptionInInitializerError
01-27 19:08:36.182: E/AndroidRuntime(12957): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onPhotoTaken(SimpleAndroidOCRActivity.java:211)
01-27 19:08:36.182: E/AndroidRuntime(12957): at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onActivityResult(SimpleAndroidOCRActivity.java:135)
01-27 19:08:36.182: E/AndroidRuntime(12957): at android.app.Activity.dispatchActivityResult(Activity.java:4649)
[...]
01-27 19:08:36.182: E/AndroidRuntime(12957): at java.lang.System.loadLibrary(System.java:535)
01-27 19:08:36.182: E/AndroidRuntime(12957): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:47)
01-27 19:08:36.182: E/AndroidRuntime(12957): ... 15 more
Please, give me the solution because this is my final project. And sorry for my english.
hey i downloaded ur project from github and imported it in eclipse
when i press the ocr button in the app, the app is force closing it and in the logcat its givin fatal exception main
plz help on this
Hello,
I’ve been training with tesseract.exe from the following Windows installation: (tesseract-ocr-setup-3.01-1.exe) in support of my Android app, which borrows from RM Theis’s work with the Tess-Two.
It seems that running tesseract.exe with the ‘batch.nochop makebox’ option (to create a box file) is the ONLY way to obtain truly accurate character positions relative to the image. Back in my Android app code, running getUTF8Text() and then getCharacters() seems to produce character boxes that are not truly aligned with the image, but are aligned with how tess interpreted the word and sentence structure in terms of whitespace. I also notice that the text output of getUTF8Text() produces correct whitespacing, whereas the character boxes output of getCharacters() evidently does not (injecting too many spaces and even splitting up words to inject a space character).
Can anyone explain this behavior? I was also wondering if I could invoke the makebox function in my Android app, so that I can display in near-realtime recognition quality feedback directly over the recognized characters themselves (using a semi-transparent Android overlay) and replacing the imaged characters with crisp artificial characters of the same font. Or if there is any other way to get those original character box positions within my app source code, I would love to know it.
Thanks!
Ted
dear gautam i just want to add selectable region in my camera plz help me through this .i am not able to seperate selectable region code
https://github.com/rmtheis/android-ocr
use this rmtheis work it has a border.
i have and error when building the ndk, this is the error: jni/../external/libjpeg/jidctfst.S: Assembler messages:
jni/../external/libjpeg/jidctfst.S:66: Error: missing ‘)’
jni/../external/libjpeg/jidctfst.S:66: Error: garbage following instruction — ` pld (r2,#0)’
jni/../external/libjpeg/jidctfst.S:259: Error: missing ‘)’
jni/../external/libjpeg/jidctfst.S:259: Error: garbage following instruction — `pld (sp,#32)’
jni/../external/libjpeg/jidctfst.S:271: Error: missing ‘)’
jni/../external/libjpeg/jidctfst.S:271: Error: garbage following instruction — `pld (ip,#32)’
/cygdrive/d/Programming/Programs/android/android-ndk-r8d/build/core/build-binary .mk:267: recipe for target `obj/local/armeabi/objs/jpeg/jidctfst.o’ failed
make: *** [obj/local/armeabi/objs/jpeg/jidctfst.o] Error 1
i already checked this file and didn’t find that missing ‘)’
can any one help ?
Hi,
I followed your instruction and advice here
I download new eclipse, new ADT and SDK, and NDK r6b.
then ndk-build, update and ant give:
Compile++ thumb : tess <= resultiterator.cpp
Compile++ thumb : tess libs/armeabi/libtess.so
confusion@confusions:~/programovani/NoSpaces/eclipceWorkspace/OCR/tess-two-master/tess-two$
.
.
.
confusion@confusions:~/programovani/NoSpaces/eclipceWorkspace/OCR/tess-two-master/tess-two$ android update project --path . --target android-10
Updated project.properties
Updated local.properties
Added file ./proguard-project.txt
confusion@confusions:~/programovani/NoSpaces/eclipceWorkspace/OCR/tess-two-master/tess-two$ ant release
.
.
.
-post-build:
release:
BUILD SUCCESSFUL
Total time: 7 seconds
and then to the eclipse and import lib, but eclipse shows that there are erros screen 🙁
and I downloaded your demo where is error because its not linked to the lib, because i can’t handle step 4.
and this is the your project properities/android screen
Can you help me or guide me some way please?
So I find out the problem, and it was stupid error.
But, is there any minimum api level? On my android 2.3.4 is going down, with -1 I think 🙂
I tried to debug the Simple Android OCR (for begginers – demo) and got “Dalvik VM[localhost:8600]” on line 208
TessBaseAPI baseApi = new TessBaseAPI();
Why? please help!
So finally, I have to work on Windows, and it is simplier 🙂
with this
http://www.pocketmagic.net/2010/08/android-ndk-for-windows-setup-guide/#.US4Cgzsqdj7
and this
http://www.jameselsey.co.uk/blogs/techblog/tesseract-ocr-on-android-is-easier-if-you-maven-ise-it-works-on-windows-too/
and Simple Android OCR working 🙂
I can’t get this to work; my NDK is not being recognized. Is it not possible to download the tess-two project itself so I can import it into Eclipse? Would save alot of trouble.
Why sometimes the recognized text is a bunch of random characters? I get this result even if there is no character in the picture. Also, I get the words right but at the start or at the end of the result show up some random characters.
@rmtheis sir is it possible to create menu screen on your OCRtest? and i would to ask if how do the translation process work on your OCR?
thank you in advance
Yes, you can add menus wherever you want. Translation uses the Google Translate API.
@rmtheis thank you so much, but there’s one thing. is there any way to use Google translate API for Free? I’ve just checked the latest version and its pricing is base on usage of character? please help.
thank you again in advance
i really appreciate your reply.
No. The Bing Translator API is an alternative that’s free up to, I think, 20M characters.
@rmtheis the alternative translate API which is the bing translator do not work anymore? i guess have no other choice buy to use the google translate API?
thank you
@rmtheis does all i need to do is put the Client id & secret key and the translation will work?
Everything works perfect, but I have one question. How can I show any message or something to inform user that searching is on progress? Now there is completly black screen during searching (generating recognizedText) and it looks like app is suspended.
I have same question 🙂
@James Esley:
Okey, I did it. But still have few questions.
Can you help me or give some advice?
I wrote this:
It is still working, but is there some other way how to pass the string to mainthread from onPostExecute, mayby via variable, I need to work with that before putting to editText.
And how can I show something like splashscreen while recognizing, lunched from onPreExecute? via acitivty with picture?
thank you for your help.
Put the recognisation into an async task (so it doesnt lock main processing thread and induce an ANR), and then display an activity indicator that says “processing…”
hi Gautam! im deo reyes, an IT student from UST, Manila, Philippines.
im currently using your tutorial on building a basic OCR app on android. I also used rmtheis’ version. I have just one error which never showed up on any web search and troubleshooting sites such as stackoverflow. here is the error:
The container ‘Android Dependencies’ references non existing library ‘C:UsersDeoDesktoptess-twotess-two.jar’
I have compiled tess-two via cygwin successfully. What could be the problem? I have a feeling it is the next set of commands, but it doesnt work on my cygwin:
android update project –path .
ant release
this is the only problem left so i could compile the project. please help asap. thank you very much 🙂
Anyone please tell the algorithm for the image processing…
Thanks Gautam and James Elsey for your experience of implement on Windows!
Glad I was of help! 🙂
I am compiling the tess-two using android NDK on windows 7 environment. I am getting following error:
[obj/local/armeabi/libgnustl_static.a] Error 1 tess-two C/C++ Problem.
Can you please help to solve this issue.
Thanks
Ajit
hello,
I have a problem. This is logcat log. Help me, please
03-07 22:13:18.394: D/AudioSystem(26104): AudioSystem::get_audio_flinger() in at 53
03-07 22:13:18.394: D/AudioSystem(26104): gLock get at 55
03-07 22:13:18.394: D/AudioSystem(26104): before defaultServiceManager() at 57
03-07 22:13:18.394: D/AudioSystem(26104): after defaultServiceManager() at 59
03-07 22:13:18.394: D/AudioSystem(26104): service got at 63
03-07 22:13:18.394: D/AudioSystem(26104): leave AudioSystem::get_audio_flinger() at 81
03-07 22:13:18.394: D/AudioSystem(26104): AudioSystem::get_audio_flinger() in at 53
03-07 22:13:18.394: D/AudioSystem(26104): gLock get at 55
03-07 22:13:18.394: D/AudioSystem(26104): leave AudioSystem::get_audio_flinger() at 81
03-07 22:13:18.444: W/MediaPlayer(26104): info/warning (1, 902)
03-07 22:13:18.464: D/LanguageCodeHelper(26104): getOcrLanguageName: eng->English
03-07 22:13:18.464: D/LanguageCodeHelper(26104): getTranslationLanguageName: es->Spanish
03-07 22:13:18.574: W/dalvikvm(26104): Exception Ljava/lang/UnsatisfiedLinkError; thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI;
03-07 22:13:18.574: D/AndroidRuntime(26104): Shutting down VM
03-07 22:13:18.574: W/dalvikvm(26104): threadid=1: thread exiting with uncaught exception (group=0x40ad0228)
03-07 22:13:18.584: E/AndroidRuntime(26104): FATAL EXCEPTION: main
03-07 22:13:18.584: E/AndroidRuntime(26104): java.lang.ExceptionInInitializerError
03-07 22:13:18.584: E/AndroidRuntime(26104): at edu.sfsu.cs.orange.ocr.CaptureActivity.initOcrEngine(CaptureActivity.java:714)
03-07 22:13:18.584: E/AndroidRuntime(26104): at edu.sfsu.cs.orange.ocr.CaptureActivity.onResume(CaptureActivity.java:371)
03-07 22:13:18.584: E/AndroidRuntime(26104): at android.app.Instrumentation.callActivityOnResume(Instrumentation.java:1236)
[...]
03-07 22:13:18.584: E/AndroidRuntime(26104): at java.lang.System.loadLibrary(System.java:535)
03-07 22:13:18.584: E/AndroidRuntime(26104): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:44)
03-07 22:13:18.584: E/AndroidRuntime(26104): ... 17 more
Hi Gautam, I was able to run your example in Windows plataform!
Now,I would like to know if there is a method to increase the accuracy of the OCR. My app is not much accurate, has a trick like distance, size of letters.. something like that?
Thanks!!
André
Hi André,
Could you please, tell me what are the steps you taken to make it run on Windows platform. How you could able to compile the library and Add without getting errors ?
Thanx !
– Nisal_M
Hi Nisal_M,
I had some problems but I was able to run. I followed this steps:
1- Install Android SDK
2- Set in the path variable the path for android command
3- Run the SDK Manager and install the Android plataform-tools
4- Download and install the ADT Plugin for Eclipse
5- Download and unzip NDK
6- Set in the path variable the path for ndk-build command (you don’t need to install Cgwin)
7- Enter in the tess-two directory and run the ndk-build command
8- Run the command:
android update project –path .
7- Import the tess-two project to Eclipse and set it like library.
8- Import the Gautam’s project and add the tess-two (now as a library).
It worked for me!
Hi, I would to use this OCR to ricognize license plates and i have a doubt: Does The tesseract try to form a word when ricognize a sequence of characters. I’m asking because my language is portuguese and i’m using eng.trainnedata and I observed that the application tries to do this? s it right?
Thanks,
André
Hi James! I was thinking about crop the image, to reduce the time of processing. Now I’ll study how do that. Thanks!
How can I set up the black/white list? Could You tell me please?
Regards,
André
Thanks James! I’ll let you know!!!
Now, I’m trying to use the por.trainneddata
I unzipped the file and I put it in the same directory (tessdata) of eng.trainneddata. After I changed the line codes to adjust. When I run the app Logcat says me that was ocurred a javaIoException. In my mobile I saw the tessadata directory and the files .trainneddata with zero size. I’m making it in a wrong way?
Thank you!
Hi Andre,
I’ve done the same for UK license plates, it was relatively easy once you set up a black/whitelist of the characters you expect.
You may also need to crop the image so you just get the number plate and it doesn’t try to OCR things in the background, like road signs etc.
I don’t have the code ready at hand, but from memory I believe you just set the black and white list on the tessBaseApi directly.
For the white list I set A-Z0-9 and for the black list I just put in a load of special characters.
Have a play with that and let us know if it improves the results, it certainly helped me (in combination with cropping)
Hi guys i have a problem with ndk-build 🙁 please help me i researched so much in internet but i didnt find solution.
My problem is in step ndk-build;
.workspace/tess-two/jni/com_googlecode_tesseract_android/Android.mk:tess: LOCAL_MODULE_FILENAME should not include file extensions
Android NDK: workspace/tess-two/jni/com_googlecode_tesseract_android/Android.mk:tess: LOCAL_MODULE_FILENAME must not contain a file extension
/android-ndk-r6-crystax-2/build/core/build-shared-library.mk:30: *** Android NDK: Aborting
Hi all!
I was able to run this app example, and now I’m trying to change the eng.trainneddata to por.trainneddata but when I do that sometimes the followed message:
Could not initialize Tesseract API with language=’por’.
Can somebody help me, please?
Thanks,
André
@rmtheis i dont know what happened but last week everything works fine. But this week when i try to export again the application and install on my device the application says. ” edu.sfsu.cs.orange.ocr” for close! Thank you for very much im advance
Hi
I would like to use this tesseract engine for reading printed equations ? Will it work on it ? Plus i am working on a Windows laptop . So is the entire process for linux or does it work for Win users too ??
Greetings,
Thank you very much for your code.
I’m trying it and I want to know if the code of the point # 5 I must include it INTO the method: protected void onPhotoTaken() when I capture the image. (The code of the other page)
thanks for your help
Greetings again.
What is: Path to the storage ??
Thanks
Hey,
I downloaded your application and when I capture the image with the camera an error pops up stating that the application stopped working, how can I solve this problem?
Thank you
I tried to build project put I found this error in step ant release
BUILD FAILED
F:\adt-bundle-windows-x86_64\adt-bundle-windows-x86_64\sdk\tools\ant\build.xml:7
10: The following error occurred while executing this line:
F:\adt-bundle-windows-x86_64\adt-bundle-windows-x86_64\sdk\tools\ant\build.xml:7
23: Error running javac.exe compiler
Total time: 3 seconds
how can I fixed this error ?
Check your java path. I was encountering the same problem. Resolved after setting JAVA_HOME path.
Hi… I can run the example in windows platform… it work form me…
now I’m in progress to make OCR app that will take picture and then translate it…
thank you Gautam for sharing… 🙂
Hi Seisy, Can you please tell me how you ran it from windows? I’m not able to do an NDK Build itself..I’m making an app which has the same module and I’m totally stuck in OCR. Would appreciate any tips and tricks for Windows
I have tried this code this works fine. But some times app terminates with “process com.test (pid 654) has died” message after executing “baseApi.getUTF8Text();”
Please help me where I am wrong.
I have same problem, and I have written to rmtheis, but no reply yet 🙁
https://github.com/rmtheis/tess-two/issues/22
Greetings, Could I combine your project with other project for scan QR Codes?
Is possible?
Thanks
Does this project work when I downloaded and import into Eclipse without any modify?
I’ve tried Robert Theis’ Android OCR application (for intermediate+), i’ve managed to avoid downloading language and orientation data by putting “osd.traineddata.zip” and “tesseract-ocr-3.01.eng.tar.zip” in assets folder. However, the uncompressing of language data went successfully but uncompressing of the orientation and script complete percentage stays at zero, and it finishes with an error about connecting to the internet, and the Log Cat shows “untar failed”. But if i restart the app it loads without any errors and the Log Cat shows that the copy of the traineddata went successfully despite it shows that it failed the first time the application started, i would be so grateful for any help :).
Thanks in advance,
Omar Mohi.
Hi i am getting this error
Could not initialize Tesseract API with language=eng!
can anyone help me soon?
How can we do the same project in Windows ? Can anyone tell me how to do an NDK Build in Windows? Looks like the whole world is using this link for their OCR,and I’m not able to do even the first step 🙁
Use cgwin building tool then u can build this project in the windows
You can use ndk builder in eclipse. If still u r having problem contact me at nauman3128@gmail.com
Thanks for responding ! I’m using NDKr8 and Eclipse Juno. I tried Cygwin also, I’m getting a .so file, Build is not completed correctly
Hey i am very thankful to you. coz you taught us a lot.
i have a question i created an by modifying the provided app in tesstwo but when i try to read more then 3-4 line of text the app crashes can you tell me the reason.
debug it using logcat
Hello,
how i can do like the picture upon , select what square will be OCR
kind regards
Dear i have one more question.
when i try to read a whole page in Emulator it works perfectly and displays the result accurately.but when i run this app on my mobile the app crashed.can you help me?
thanks for your tutorial. I am trying to implement this one. I try to change the capturing image process with image which I uploaded. And, it’s not success. So, what is your problem in your opinion? Do this library only can recognize one line word picture?
i m having a problem,whenever i run my ocr application the emulator stops on a white screen and doesnot display any my layout..
hey hw r u? i am facing a problem while running ur simple android ocr projct https://github.com/GautamGupta/Simple-Android-OCR
the problem is when even i take a picture it asks in an alert box Done or retake..i click on done then it generates an error saying the application hs stopped unexpectedly…
Please help me..
I’m also having the same problem. The app gets closed after the picture is taken. Can anybody help to rectify this error?
Anyone can help me, how to do an NDK Build in Windows?. This is the main task, anyone can tell me the procedure clearly for NDK build on window?..
Use the cgwin building tool. setup the path for NDK jar file. Then u can build.
You can use ndk builder in eclipse. If still u r having problem contact me at nauman3128@gmail.com
@ DHarshana and Nauman Thanks.successfully i completed NDK buid using windows itself. Its working. But character recognition using this OCR is giving 50 % output only. wat i have to do for improve this?
Good article. Keep it up.
Error Shows.
import com.googlecode.tesseract.android.TessBaseAPI
TessBaseAPI
Please help me
did u build it first?
Thanks for this post.settin up everythin was very straightforward for me.i have a runnin project but the text is sort of garbage.I just want to read 12digits serial numbers.is there a way to train mine to just 0-9.nunerals only and specified font?.thanks
do anyone have idea of image processing for optimized results? if so please help me in that.
Successfully i completed NDK buid using windows itself. Its working. But character recognition using this OCR is giving 50 % output only. wat i have to do for improve this?
Here is the same problem! Although everything is ok, result is not perfect.
How can I repair that?
I have successfully builed the NDK in windows, using cmd. no issues at all/ set NDK and SDK paths correctly. no issues with that too. after i build NDK and trying to execute
android update project –path .
gives an error – ‘the project either have no target set or the target is invalid ‘
somebody please help me with this. thank you !
try this on windows android.bat update project -t 8 -p .
it did not work for the value ‘8’. but for the value ‘1’.
the result is
“updated project.properties
added file \proguard-project.txt”
is this the result i should be expecting ? thnx
please email me directly to email2mefrancis@gmail.com. or searc me on fb usin this email.
Same problem here!
You can type “android list target” to see those available android targets on your machine. Then, specify the desired target_id to “android.bat update project -t target_id -p .”.
When i Go to the eclipse and try to set the tess-two as the library. The library is now shown in there. i have checked the IsLibrary too. still nothing display as a library to select. please help me
hi
the library es perfecto , but the TextView in top left not show info in live camera , before capture image
pleas help
I am trying to develop the OCR to identify equations.
I found a traineddata file that is ‘equ.traineddata’
Can I use both ‘eng’ and ‘equ’ both tessdata to my app ?
if so, how ??
thnx
How to whitelist all blackspace??
Thank you so much Gautam. This blog helped me a alot
did anyone get 100% result? if anyone, please help me
Hi all
I have imported Simple Android OCR application (complete project) , all work fine when tested on my phone but the output text doesn’t fit the text in the photo at all… why is this happening?
Hi,
awesome tutorial, but I think
bitmap = bitmap.copy(Bitmap.Config.ARGB_8888, true);
has to be outside of the if-clause (also executed, when no rotation is required)
and maybe you should include https://gaut.am/making-an-ocr-android-app-using-tesseract/#comment-61624 in your post, it helped me a lot.
Nikolas
You’re right. 🙂 Fixed!
http://stackoverflow.com/questions/17556855/tesseract-on-android-ocr-android-app
i have posted my problem on the link given above.. please please help me in solving it 🙂 .. akshay
Hi Gautam,
I am trying to follow your tutorial (thanks by the way for this, there is not much documentation out there about tesseract). I encounter some issues at the end of the compilation:
$ ndk-build
Install : liblept.so => libs/armeabi/liblept.so
Compile++ thumb : tess <= permdawg.cpp
jni/com_googlecode_tesseract_android/src/dict/permdawg.cpp: In member function 'void tesseract::Dict::go_deeper_dawg_fxn(char const*, const BLOB_CHOICE_LIST_VECTOR&, int, const CHAR_FRAGMENT_INFO*, bool, WERD_CHOICE*, float*, float*, WERD_CHOICE*, int*, void*)':
jni/com_googlecode_tesseract_android/src/dict/permdawg.cpp:208:62: error: format not a string literal and no format arguments [-Werror=format-security]
cc1plus: some warnings being treated as errors
make: *** [obj/local/armeabi/objs/tess/src/dict/permdawg.o] Error 1
And I have lots of other warnings during compilation, such as:
Compile++ thumb : lept <= readfile.cpp
jni/com_googlecode_leptonica_android/readfile.cpp: In function 'jint Java_com_googlecode_leptonica_android_ReadFile_nativeReadFiles(JNIEnv*, jclass, jstring, jstring)':
jni/com_googlecode_leptonica_android/readfile.cpp:103:12: warning: converting to non-pointer type 'int' from NULL [-Wconversion-null]
jni/com_googlecode_leptonica_android/readfile.cpp:109:12: warning: converting to non-pointer type 'int' from NULL [-Wconversion-null]
jni/com_googlecode_leptonica_android/readfile.cpp: In function 'jint Java_com_googlecode_leptonica_android_ReadFile_nativeReadFile(JNIEnv*, jclass, jstring)':
jni/com_googlecode_leptonica_android/readfile.cpp:127:12: warning: converting to non-pointer type 'int' from NULL [-Wconversion-null]
Any idea of how to solve this? I have a fresh download and install of ADT with NDK from less than a week and working on a MacBook.
Config:
$ uname -a
Darwin MacBook-XXX.local 12.4.0 Darwin Kernel Version 12.4.0: Wed May 1 17:57:12 PDT 2013; root:xnu-2050.24.15~1/RELEASE_X86_64 x86_64
$ ndk-build -v
GNU Make 3.81
Copyright (C) 2006 Free Software Foundation, Inc.
This is free software; see the source for copying conditions.
There is NO warranty; not even for MERCHANTABILITY or FITNESS FOR A
PARTICULAR PURPOSE.
This program built for x86_64-apple-darwin
$ echo $PATH
/opt/local/bin:/opt/local/sbin:/usr/bin:/bin:/usr/sbin:/sbin:/usr/local/bin:/Users/christophe/Documents/ADT/ndk:/Users/christophe/Documents/ADT/sdk/tools/:/Users/christophe/Documents/ADT/sdk/platform-tools/
There is an error on tess-two project, at line 208, file permdawg.cpp :
jni/com_googlecode_tesseract_android/src/dict/permdawg.cpp:208:62: error: format not a string literal and no format
Are we supposed to correct such errors? I have not seen anything about this case on the forums. Isn’t it more related to the compiler or system settings?
Thanks for pointing this out. This problem was introduced with NDK v9. I committed a fix, so please pull the latest tess-two code and try the build again.
@rmtheis thanks… 🙂
I had completed all the step mentioned here to get the out put ..
but unfortunately an exception occurred after taking the photo…
so i made little bit change in my application and tested with a picture that manually pushed by me to the sdcard, when i running in emulator shows the following exception
E/AndroidRuntime(3275): java.lang.ExceptionInInitializerError
08- at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.onPhotoTaken(SimpleAndroidOCRActivity.java:212)
08- at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity.startCameraActivity(SimpleAndroidOCRActivity.java:122)
08- at com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity$ButtonClickHandler.onClick(SimpleAndroidOCRActivity.java:112)
08- at android.view.View.performClick(View.java:4204)
...
08- at java.lang.System.loadLibrary(System.java:535)
08 at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:45)
Gautam, I followed all the steps in your tutorial, and everything went according to plan. I successfully imported the tess-two library in eclipse, made it a library project and imported it into my project. But, at runtime, when I try to execute:
baseApi.init(path, lang);
I get an exception: ljava/lang/unsatisfiedlinkerror thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI
can you please help me overcome this issue…
I’m developing a simple OCR receipt scanner, and I’m working on windows8.
Dear Gautam/rmtheis,
I successfully integrated your advanced OCR. I need this app to identify few certain symbols that are used in mathematics such as pi and sigma. I have successfully followed the instruction of how to train a language in google groups.
The problem I have is even I trained those symbols, they won’t appear in the OCR as it is. for an example if I scan the pi symbol, the OCR do not display the symbols as it is but it shows the corresponding letter of the keyboard which I used in the font.
what would cause this problem ?
thank you !
I have imported Simple Android OCR application (complete project) , all work fine when tested on my phone but the output text doesn’t fit the text in the photo at all… why is this happening?
I tried your basic as well as intermediate apps you have shared. The basic one doesn’t give accurate results but the intermediate one was giving a better output. Camera focus is having some problems some times in your intermediate app.
But my question is that i am trying to build an Image to Text Converter using a Cloud(Server). Would you help me with it as i have no idea about cloud computing and the 2nd problem i am facing is that there are going to be diagrams too in the document the app will be converting. How to get the diagram out of it.
Hi gautam,
This is really a nice tutorial for beginners. Can you tell me what can i do for multiple words or text-lines. When i use huge amount words of image, the program-me crashes immediately.
Thanks.
Hi, how I can detect orientation of text to auto adjust image before OCR I wrote the next line
baseApi.setPageSegMode(TessBaseAPI.PageSegMode.PSM_AUTO_OSD);
and put theosd.trainddata
file in the tessdata folder but it didn’t work. Please respond as soon as you can?how to solve
could not initialize tesseract api with language=eng
Hi there,
if I try to do TessBaseApi api = new TessBaseApi(); my application crashes.
Does anyone know why?
Thank you in advance.
Greetings
You can clean project to rebuild it, that will prevent exception like “couldn’t load lept from loader dalvik”.
I seem to have a problem running my application. In particular, loading the libraries. I get the following error:
E/AndroidRuntime(11472): Caused by: java.lang.UnsatisfiedLinkError: Couldn’t load lept: findLibrary returned null
I have tried cleaning the project and had no results.
Any ideas?
Hey Gautam. Thank you so much for this tutorial. I’m a newbie in android and this tutorial is really what I’m looking for. I have a challenge with building the tess-two project. I can’t seem to get the commands to work. I keep getting the error ‘no target set’ on cmd. I’m not sure whether I should write them one at a time or all of them together and whether to use cygwin or cmd on Windows vista. Please anyone help point me in the right direction.
I think, You have to give Android target Version no. ( i.e. Android Max version).
Hey Gautam. I tried to install but ran into difficulties. I’m using Windows and would appreciate some help:
-Downloaded and extracted NDK
-Downloaded tess-two as a ZIP file from Github, extracted it.
-Using CMD I navigated to the tess-two folder, and ran ndk-build from inside the folder. It executed the script, which took about 15 minutes.
This is now where I’m at. Tried to follow the next step, which is “android update project –path” CMD tells me “android” is not a recognized command. I thought it might be Windows, so I got cygwin and tried the same thing – it tells me “-bash: android: command not found”
Next I tried to use Eclipse to build tess-two. I set NDK path as the NDK builder. Then I tried to import the tess-two project, but immediately I got the following errors: “Project has no project.properties file! Edit the project properties to set one.”
I decided to plough on. I tried to set a new Builder for tess-two using NDK (I set the location as the path for ndk-build and set the working directory as $(workspace)/tess-two.
However upon trying to build tess-two, it gave me the following build error:
“Unable to resolve target ‘android-8′”. It then refuses to build.
Where am I going wrong?
Your “android” command was not found because you need to add the Android SDK to your PATH.
Hi Gautam. I followed all the steps successfully. Everything works fine but I am not getting the exact text as the image contains. Please help me in this issue. Every time it returns something new or unique character. Thanks in advance.
Hey Gautam, I cannot translate TRADITIONAL_CHINESE, zh_TW, to SIMPLIFIED_CHINESE, zh_CN. The TRADITIONAL_CHINESE can be scanned and show in screen but the output is remain in TRADITIONAL_CHINESE. The translation problem only occurs in zh_TW to zh_CN.
Guys I need help, I don’t know how to build it, there was no ndk-build.cmd inside the tess-two folder
After installing the NDK, you can go in your tess folder until ../tess-two as specified by Gautam.
With command line put: “ndk-build Path-of-NDK-location” while working for windows command line. After building, go with command line in your SDK folder. (You will see some androids tools.). Type: “android update project -t 1 -p path-of-Tess-project”
Finally there is the step with ant release but for now it failed for me.
Hi back…correction being in your folder of tess 2 : put : /ndk-build, same thing for ant release : /ant release. Hope it will help. It is all right on Wndows command line in this way.
erratum : “/ndk-build”
I’m stuck at the step “ant release”
-BASH: ant: command not found
Downlaod ant, extract the zip and set system variables to ant/bin folder. Close your command prompt and open it again, type ant -version
How to determine if the character belongs to the rectangle box bound?
Nice blog. Any ideas for getting better results using Tesseract? Please suggest any ideas on pre-processing the image before providing it to Tesseract for recognition..
Hi, I was away for a bit. I manged to solve my problem. Simply used eclipse and set it as library then it produced a jar file with accompanying classes
Hello, I am working on a Sencha Touch 2 + Phonegap 3 app and intend to use tess-two as an ocr plugin in phonegap. I have successfully build tess-two. However, the folder is about 400MB++ (way too big), which folder specifically should I copy for my project. Should it be the bin or libs or jni? Any feedback will be greatly appreciated
same problem here my tess-two folder is over 400 MB,
did you solve it?
Guys, I need help. I can finish step 1 to 3. But I’ve problem that I can’t understand it in step 4 and 5.
the script in step 4 and 5, where it should be placed? Can anyone explain it, or give the whole script because I’ve stuck in that part.
Thank you in advance.
Best Regard.
I don’t know why but I included the tess-two library and somehow tried to build it but failed (it seems to be built though). And then first I ran it on an emulator, it says SD card was corrupted/unavailable. After I solved the SD card problem, it just popped out a black screen and said OCR Test has stopped. Please help me
Leo, could you resolve the problem “OCR Test has stopped”?
I have done all your steps but couldn’t get the right result can you please tell me what is the problem.
The app works fine with English traineddata(eng.traineddata). But i have to use it for French. i put the fra.traineddata file in assets/tessdata folder but it wont work. the error it shows:
Installation error: INSTALL_FAILED_INSUFFICIENT_STORAGE
though there is sufficient storage on my phone.
Please help.
Hi Gautam, i moved on all steps and it build success fully but i get these error pls help me
ActivityManager: Starting: Intent { act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] cmp=com.example.rexdroid/.RexDroidOCRActivity }
This is the error i getting pls help me to solve it……
[2014-03-19 11:44:17 – Simple-Android-OCR] Installing Simple-Android-OCR.apk…
[2014-03-19 11:44:37 – Simple-Android-OCR] Success!
[2014-03-19 11:44:37 – tess-two] Could not find tess-two.apk!
[2014-03-19 11:44:37 – Simple-Android-OCR] Starting activity com.datumdroid.android.ocr.simple.SimpleAndroidOCRActivity on device emulator-5554
[2014-03-19 11:44:38 – Simple-Android-OCR] ActivityManager: Starting: Intent { act=android.intent.action.MAIN cat=[android.intent.category.LAUNCHER] cmp=com.datumdroid.android.ocr.simple/.SimpleAndroidOCRActivity }
Check my blog here for an easy solution. http://androidtesstwo.blogspot.com/
After installing all s/w I am facing problem in step 2.
‘ndk-build’ is not recognize as an internal or external command, operable program or batch file.
I have successfully completed all the steps as mentioned above.but,now i am getting error given below:
tess-two] Failed to create BuildConfig class
Simple-Android-OCR] Failed to create BuildConfig class
tess-two] Failed to create BuildConfig class
Simple-Android-OCR] Failed to create BuildConfig class
Please Help me.
Hello Gautam,
Great job.
I have a question for you. Do you think this OCR reader could be used in Google Glass? I want to implement an app using OCR reader for Google glass. Any thoughts?
Thanks,
Pramod
Hello,
I really enjoyed your tutorial, I used Tesseract in a school project and it worked well !
I did a french translation of the installation on my blog, if you want to add to translation list 😉
Thanks
Great tutorial, I can’t thank you enough!
Wow… Superb job Gautam.. I done importing libraries like test-two and also assigned path to ndk build successfully… I solved all the errors.. But a problem, When I am trying to run, it shows an error message after captured image i.e, “Unfortunately your application has been stopped” .. I can’t find whats the mistake. Help me to run this app without any problem..
I have same problem , if you solve it help me please?
Same happens to me, after taking the picture the app is stopped and there is this error in the LogCat:
07-03 00:38:56.281: E/AndroidRuntime(3108): FATAL EXCEPTION: main
07-03 00:38:56.281: E/AndroidRuntime(3108): Process: com.datumdroid.android.ocr.simple, PID: 3108
07-03 00:38:56.281: E/AndroidRuntime(3108): java.lang.UnsatisfiedLinkError: Couldn’t load lept from loader dalvik.system.PathClassLoader[DexPathList[[zip file “/data/app/com.datumdroid.android.ocr.simple-1.apk”],nativeLibraryDirectories=[/data/app-lib/com.datumdroid.android.ocr.simple-1, /vendor/lib, /system/lib]]]: findLibrary returned null
Hi there!
Is anybody who had same problem as me with Leptonica library?
When I call findSkew on my image, it returns 0.0..:-(
Float s = Skew.findSkew(ReadFile.readBitmap(image));
I tried both methods…
Thanks!
Hi Gautam,i really admired about your OCR Blog, such a really nice one.
but i don’t know it does not work for me in eclipse exactly of your code.
can you guide me if i am on wrong somewhere. and also actally i don’t want to store image in sd card just directly read from image without save to sd card and bind to the EdittextBox. please bro help me an out from this.
I solved all the errors.. But a problem, When I am trying to run, it shows an error message after captured image press ok (ok,retake,cancel) it crash application i.e, “Unfortunately your application has been stopped” .. I can’t find whats the mistake. Help me to run this app without any problem..
sir I just want to ask I encounter this while i’m updating the android update project –path it says ” it seems that there are sub-projects. if you want to update them please use the –subprojects parameter”. How would I use the subproject parameter.. please help me I am a newbie in android.. thank you
Hello, at the beginning :
cd /tess-two
ndk-build
android update project –path .
ant release
what does the android update project –path mean?
I’m using windows, and can’t use those commands on cmd.
I have that same problem ‘android’ is not recognized as a command
Hi Mr.Gautam!
I have used this Tesseract OCR App. It works perfectly.
Thanks for publishing this App. I need to implement this in my app. Where i need to read the characters in an already saved JPEG image by OCR. In your coding where exactly, you are passing the image to read by the OCR. Kindly suggest any idea to call OCR by giving URI of the image.
Thanks in advance. Looking forward for your valuable reply.
java.lang.UnsatisfiedLinkError: Couldn’t load lept from loader
dalvik.system.PathClassLoader[DexPathList[[zip file “/data/app/edu.sfsu.cs.orange.ocr-1.apk”], nativeLibraryDirectories=[/data/app-lib/edu.sfsu.cs.orange.ocr-1, /vendor/lib, /system/lib]]]: findLibrary returned null
@Sharafkar @Sha @Demon
You have to follow the instructions to *build* tess-two as well.
Caused by: java.lang.UnsatisfiedLinkError: Couldn’t load lept from loader dalvik.system.PathClassLoader[dexPath=/data/app/edu.sfsu.cs.orange.ocr-1.apk,libraryPath=/data/app-lib/edu.sfsu.cs.orange.ocr-1]: findLibrary returned null
at java.lang.Runtime.loadLibrary(Runtime.java:365)
at java.lang.System.loadLibrary(System.java:535)
Hi Mr. Gautam,
I followed your Instructions
First I imported Tess-Two from github: https://github.com/rmtheis/tess-two
And linked it to my project https://github.com/GautamGupta/Simple-Android-OCR
The app compiles and runs fine. But after clicking an image when I hit done it crashes.
And I think the problem is in here:
TessBaseAPI baseApi = new TessBaseAPI();
baseApi.setDebug(true);
baseApi.init(DATA_PATH, lang);
baseApi.setImage(bitmap);
String recognizedText = baseApi.getUTF8Text();
baseApi.end();
Hi, Thanks for this tutorial.
I have a problem when I get to the baseApi.init(….) line. it stops and the log shows ” UnsatisfiedLinkError : cannot load library : reloc library[1306] : 1683 cannot locate ‘rand’ … ”
Does that mean the building of the tess two went wrong ? if so, I kept redoing it and still the same error. What should I do ?
Thanks 🙂
@Mohamed
There’s a comment from an NDK team member on a related bug tracker issue that says you need to “unzip ndk64* on top of ndk32* to get all API levels for 32-bit development” because they changed some things around in NDK r10.
Please open an issue on the tess-two project if you continue to see the problem.
nice job , but i have a problem
i get this exception whenever it comes to initializing tesseract
“Data path must contain subfolder tessdata ”
but i’m sure the path is correct and the .trained file is there
i’m familiar with tesseract – Emgu Cv C# – and things were so much easier
i’m new to Android So your help will be appreciated
Hi, i also have same problem like you now. Have you find out? Can you tell me how to solve it? Thanks 🙂
Hello thanks for the tutorial, recently i started making an ocr app and its complete but i have a problem the text recognized are not accurate can you please give me a solution to this.
Thanks for your time..
George
Helpede alot. I am able to get it working for my app.. Thanks alot
Im working on windows 7 PC. when I run ‘ant release’ on command prompt it returns
C:\eclipseworkspace\tess-two-master\tess-two>ant realease
Buildfile: C:\eclipseworkspace\tess-two-master\tess-two\build.xml
BUILD FAILED
Target “realease” does not exist in the project “tess-two”.
Total time: 2 seconds
Be sure you spell “release” correctly.
I want to crop the image and starighten it autmatically. like the work done in this app https://play.google.com/store/apps/details?id=com.cyberlink.yousnap&hl=en
i am trying to use the native lib in my Android project…
Give an idea regrading OpenCV native library to use in my face recognition project and your project Optical character recognition ideas in my another app… Please give me some initial steps so that i’ll be able work out with my project..
kindly reply
Hi Mr.Gautam
I have some problem in step 5. I’m trying to create rotation when I compile it has not a problem but the picture can not rotate. Please give me and idea. Thank you.
Hello 🙂
I can not execute this
“About updating PATH – You need to update your PATH variable for the commands to function, otherwise you would see a command not found error. For Android SDK, add the location of the SDK’s tools and platform-tools directories to your PATH environment variable. For Android NDK, use the same process to add the android-ndk directory to the PATH variable.”
Please help me
I was having a lot of trouble getting this to compile in Android Studio (especially incorporating the Tessaract library into the app). But this link helped a lot:
https://coderwall.com/p/eurvaq/tesseract-with-andoird-and-gradle
Worked fine.. Thanks for ur post.
Hello!
I’ve followed your steps and eventually I build the tess-two project successfully (on windows 8.1 btw ), but when I try to run the app it doesn’t work. It says: “Could not find Simple-Android-OCR.apk!” Any suggestions?
Thanks!
Hello your post is really awesome i have successfully completed all the steps and no error in eclipse but when i run application i suddenly shows an error message that simple android OCR unfortunately stopped now what to do any suggestions please reply me as faster as possible…
My application is running on mobile phone but it is not recognizing text properly…!!
Any suggestions what to do for it…???
Any One help me to make this application for any other two languages?
Hey!! I am getting an error saying allheaders.h missing
link :- https://stackoverflow.com/questions/27958943/missing-allheaders-h-in-android-studio-project
HI Im just a starter in developing an android app. Is this efffective in Android Studio ? Ty
Android Studio doesn’t have real NDK support, so personally I would give it a go in Eclipse.
I am trying to include the tesseract OCR for a school project, but am getting Problems with the NDK. I am using NDK r10d on a mac mostly because I can not find r7c. So my Question is, what is the latest NDK compatible for this OCR?
Here is my Error maybe that would help.
Error:Execution failed for task ‘:tesstwo:compileReleaseNdk’.
> com.android.ide.common.internal.LoggedErrorException: Failed to run command:
/Developer/adt-bundle-mac-x86_64-20140702/android-ndk-r10d/ndk-build NDK_PROJECT_PATH=null APP_BUILD_SCRIPT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/Android.mk APP_PLATFORM=android-8 NDK_OUT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/obj NDK_LIBS_OUT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/lib APP_ABI=all
Error Code:
2
Output:
In file included from /Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/src/main/jni/com_googlecode_leptonica_android/box.cpp:17:0:
/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/src/main/jni/com_googlecode_leptonica_android/common.h:22:24: fatal error: allheaders.h: No such file or directory
#include
^
compilation terminated.
make: *** [/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/obj/local/arm64-v8a/objs/tesstwo//Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/src/main/jni/com_googlecode_leptonica_android/box.o] Error 1
the path to the NDK should be correct…
Thanks for your help,
Adrian
So it’s me again, it seems like a file (allheaders.h) is missing, thats why I thought, that the NDK r10d 64 bit might not workout. Others had problems using a other NDK as suggested. The oldest NDK I can download is the 10c, by changing download link manually as suggested in various Blogs.
I am developing on Android Studio, as seen in the error.
So far the Blog was great
Thanks again,
Adrian
It’s not a problem with NDK r10d — I can build with that version of the NDK and all tests pass. You’re seeing a problem with importing the library project into Android Studio.
So again it is me. I have now used NDK r7c and got a new Error. In the comments above were similar problems but under windows, and the answer seemed to be use Ubuntu.
So despite I may be starting to seem like a spammer, I would like to here your advice to this error:
Error:Execution failed for task ‘:tesstwo:compileReleaseNdk’.
> com.android.ide.common.internal.LoggedErrorException: Failed to run command:
/Developer/android-ndk-r7c/ndk-build NDK_PROJECT_PATH=null APP_BUILD_SCRIPT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/Android.mk APP_PLATFORM=android-8 NDK_OUT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/obj NDK_LIBS_OUT=/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/lib APP_ABI=all
Error Code:
2
Output:
make: *** No rule to make target `/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release//Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/src/main/jni/com_googlecode_leptonica_android/box.cpp’, needed by `/Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/build/intermediates/ndk/release/obj/local/armeabi/objs/tesstwo//Users/myName/AndroidStudioProjects/TextErkennung2/tesstwo/src/main/jni/com_googlecode_leptonica_android/box.o’. Stop.
Hope to here an Answer soon (is a school project and deadline is coming close – maybe I underestimated this)
regards,
Adrian
hello sir adrian, i am a beginner and i just wanna know if it is fit in windows? and where should i build it ? in cmd ? thanks in advanced.
@Jay
As said I am using Mac OS X and Android Studio to develop. But Android Studio seems to have difficulty with the Library (as Robert Theis said). Eclipse is used in this tutorial so I can only recommend it, although I am having trouble with Eclipse and Android.
Building my project with Tess-Two hasn’t worked yet so I am concentrating on other parts right now.
As said I am not using Windows you might check other comments here, how they approached it. Or if that doesn’t help out check other tuts that are linked on this site.
Regards,
Adrian
hi i looking to make a project using ocr which recognizes equations and solves them. I have never used ocr. i would like to to know if i can do this and if yes how so?
Hello, im a student from cuba and i want to use tesseract library to develop an app that use the camera to recover data from a picture number and text and i dont have much internet acces i need help with that. Im using eclipse for the coding. I need to know how to use tesseract. Thanks
I got following error can anyone help me?
02-04 12:25:23.954: E/AndroidRuntime(10024): FATAL EXCEPTION: main
02-04 12:25:23.954: E/AndroidRuntime(10024): java.lang.UnsatisfiedLinkError: Couldn't load lept from loader dalvik.system.PathClassLoader[DexPathList[[zip file "/data/app/edu.sfsu.cs.orange.ocr-2.apk"],nativeLibraryDirectories=[/data/app-lib/edu.sfsu.cs.orange.ocr-2, /vendor/lib, /system/lib]]]: findLibrary returned null
02-04 12:25:23.954: E/AndroidRuntime(10024): at java.lang.Runtime.loadLibrary(Runtime.java:355)
02-04 12:25:23.954: E/AndroidRuntime(10024): at java.lang.System.loadLibrary(System.java:525)
02-04 12:25:23.954: E/AndroidRuntime(10024): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:43)
02-04 12:25:23.954: E/AndroidRuntime(10024): at edu.sfsu.cs.orange.ocr.CaptureActivity.initOcrEngine(CaptureActivity.java:711)
02-04 12:25:23.954: E/AndroidRuntime(10024): at edu.sfsu.cs.orange.ocr.CaptureActivity.onResume(CaptureActivity.java:368)
02-04 12:25:23.954: E/AndroidRuntime(10024): at android.app.Instrumentation.callActivityOnResume(Instrumentation.java:1192)
02-04 12:25:23.954: E/AndroidRuntime(10024): at com.lbe.security.service.core.client.b.x.callActivityOnResume(Unknown Source)
02-04 12:25:23.954: E/AndroidRuntime(10024): at android.app.Activity.performResume(Activity.java:5213)
02-04 12:25:23.954: E/AndroidRuntime(10024): at miui.dexspy.DexspyInstaller.invokeOriginalMethodNative(Native Method)
...
Hi Nayank,
Were you able to fix this error ? Even I’m getting the same error
How can I implement OCR for other languages? Which changes is required in Tess-Two project?
I want to add user word list for get more accuracy in tess-two or my project …….but i dont know how , plz help me
@Sunil, give me your email address I will send you the solution, this error is occurred due to the project setup problem.
sunilmathewsoman@gmail.com.
sunilmathewsoman@gmail.com
Hi Nayank, please send to me too!
mtpoppleton@gmail.com
Does anyone know how to implement the “torch_button” feature? I see it commented out but I’m not sure how to write the function to turn it on without the app crashing.
I am using this demo but give me some time Fatal signal 11 (SIGSEGV) at 0x00000000 (code=1), thread 4197 . I am not getting solution of this error. can you explain why i am getting this error.
hey……hie gautam.. i followed the tutorial and it helped me a lot…but….it runs correctly at the first attempt…After my first attempt i have to re-install tha apk file and run again…otherwise it prints the garbage value..
.can you provide any solution to this….?
Hi,
That was really awesome!
I’m trying with something like this. Can you tell me how to get the baseline or Rect from the Tesseract?
platform – android“`
Hi. After giving up a bunch of times, I am finally able to run both the apps. I can understand the Simple Android OCR source, but is there a guide to the intermediate+ level Android OCR app?
Thanks.
03-30 15:09:53.358 31833-31833/com.example.dellpc.material2 I/Tesseract(native)﹕ Attempting Init() with dir=/storage/emulated/0/BTP/, lang=dut11
03-30 15:09:53.359 31833-31833/com.example.dellpc.material2 A/libc﹕ Fatal signal 11 (SIGSEGV) at 0x00000000 (code=1), thread 31833 (ellpc.material2)
i am getting this error and stopping my app when i try to native tessbaseapi init function
Hi,
That’s a very nice work. Am working on a school project. Android app which will read numbers on any surface via camera and give an option to either call or send sms to the scanned number.
Am counting on you guys.
Pls I need help on this.
ASAP.
Regards.
when I import android-ocr-master Or simple-android-ocr-master in “Android Studio” make this error
* Project OCRTest:E:\1-Our Project\android-ocr-master\android\project.properties:
Library reference ..\..\tess\tess-two could not be found
how i can fix it ?
Did you add tess-two as a library if you did you have to make it as a module dependency to your main project. This link might be helpful https://coderwall.com/p/eurvaq/tesseract-with-andoird-and-gradle
Hi, I wonder what kind of images OCR works, what are the allowed extensions or if a the results are better
Hi Gautam
Thanks for this article.
Please is it possible to use Tesseract with Xamarin?
I have been developing with Xamarin but I now need to build mobile applications with OCR capability.
Hi guys, I need a help~ I stucked in the step 2 .
I dont know how it actually working.
cd /tess-two
ndk-build
android update project –path .
ant release
Can you guys tell me in details for each command line ?Please =(
I am working on an app that requires OCR capabilities but facing difficulties with running the app,
04-15 13:02:34.612: E/AndroidRuntime(7410): java.lang.NoClassDefFoundError: Failed resolution of: Lcom/googlecode/tesseract/android/TessBaseAPI;
searched the web since 4 hours, did not find a appropriate solution, can any one help me with this
thanks
did you properly build Tess-Two and imported..?
and don’t forget to give reference of Tess-two to your project.
hi thank you so much for this tutorial , you literally saved me !!! but i have one problem ! i tried to extract only the code that contains the selectable region while capturing from the intermediate+ project but i couldn’t .. any help please ! i don’t need full answer , just guide me to the right path :p
For ANDROID STUDIO developers, here’s what I did on my Windows 8.1 machine.
1) Download and install the ndk from here https://developer.android.com/tools/sdk/ndk/index.html. I had some trouble with its path in following steps so i put it in “C:\”.
2) Add that path to environment variables of the system (eg: “C:\android_ndk_r10d”) and then reboot so your machine can find it.
3) Download “tess-two-master” from here https://github.com/rmtheis/tess-two, extract it (for example in “C:\”) and rename it in “tess”.
4) Open “tess” folder and then open “tess-two” folder. Click on a blank space while pressing the shift button and select “Open command window here”.
5) Write “ndk-build” and wait until it completes (about 20min).
6) Go back in the parent folder, select “eyes-two” folder and again click on a blank space while pressing the shift button in order to open the command window.
7) Write “ndk-build” and wait.
8) Write “android update project –target 1 –path C:\tess\tess-two”. Of course I assume your “tess” folder is located in “C:\”
9) Write “ant release”. IF IT COMPLAINS go where you change your system environment variables as you did in step 2 and ADD a new variable with name “JAVA_HOME” and value the path to your jdk (eg: “C:\Program Files\Java\jdk1.8.0_40”)
10) Open a completely new Android Studio project and follow these instructions https://coderwall.com/p/eurvaq/tesseract-with-andoird-and-gradle from section “Configure tess-two with gradle” but for safety don’t delete any folder or file even if he suggests to do so.
I had some trouble with “build.gradle” file in “libraries\tess-two” directory, but it is sufficient to change some value on it. In my case I have:
“classpath ‘com.android.tools.build:gradle:0.14.0′” instead of “classpath ‘com.android.tools.build:gradle:0.9.+’ ”
and
” compileSdkVersion 21
buildToolsVersion “21.0.2”
defaultConfig {
minSdkVersion 15
targetSdkVersion 21
} ”
instead of
” compileSdkVersion 19
buildToolsVersion “19.0.3”
defaultConfig {
minSdkVersion 8
targetSdkVersion 19}”.
Note that the last step means you have to go in “File -> Project Structure -> Select a module from the left subwindow -> Dependencies (last tab) ->Press the green “+” on your right -> Module Dependency -> OK”
11) Download this project https://github.com/GautamGupta/Simple-Android-OCR and copy&paste in your new project the code in these files: “SimpleAndroidOCRActivity.java”, “main.xml”, “strings.xml”. Of course your files may have different names (in my case “MainActivity.java”, “activity_main.xml”, “strings.xml”) so some renaming in the code may be necessary.
Also open your “AndroidManifest.xml” and add at the end (but before “/manifest”) what you find between “/application” and “/manifest” in the just downloaded “AndroidManifest.xml” (it means that you have to add “uses-permissions” and “uses-feature” tag).
12) Download from here https://code.google.com/p/tesseract-ocr/downloads/list the file in the language you prefer (eg: tesseract-ocr-3.02.eng.tar.gz ), extract it and locate the file “yourLanguage.traineddata” (eg: “eng.traineddata”). Forget for a moment your Android Studio IDE, open the folder of your project and go in “app–>src–>main”. Create here a new folder and name it “assets”. Open it and create another folder named “tessdata”. Put there your .traineddata file.
13) Hope it was helpful, I obtained this after weeks of googling during free time, sometimes reaching page #10+. If I forgot something please forgive me and please reply this post without hesitation. Since I started android programming only 2 months ago I won’t be able to answer your questions. Forgive my bad english too.
Ciao belli!
Awesome! Give my android-ocr project a try too!
Thanks a lot Matz.
So if I put the .traineddata file in main/assets/tessdata, what should be the code here? :
baseApi.init(DATA_PATH, lang);
// Eg. baseApi.init(“/mnt/sdcard/tesseract/tessdata/eng.traineddata”, “eng”);
thanks in advance,
Quentin
Thanks Matz. worked. Can I integrate a more refined character reader? This one is a bit confused. Or let me ask, how can I refine the library to read particular text? like certain text on a notice. Like particular fonts or patterns
You are a life saver, thank you
Hi, thanks for your tutorial.
I got an error at step 5: “host awk tool is outdated. please define NDK_HOST_AWK to point to Gawk or Nawk!” I’ve tried to delete the outdated awk.exe file as instructed by others but no progress. Have you met such problems?
Hey could you clarify step 11 for me? Didnt quite get that
Also if Someone could tell me what all to replace in the 11th step. that would be awsome!
could you please explain the 8 step ” android update project –target 1 –path C:\tess\tess-two”??? because iv got error in cmd
Hi! Im following all steps, but I get this gradle error…:
Error:Configuration with name ‘default’ not found.
Any thoughts?
How can I do this step? 8) Write “android update project –target 1 –path C:\tess\tess-two”. Of course I assume your “tess” folder is located in “C:\”
it gives me an error saying android is not recognized as an internal or external command. I put the sdk/tools and platform tools directory on the path environment variables but still it has that error. hope someone can help me. thank you
Hello. Please, clarify something to me, do I need to have cygwin installed or something? when I run the ndk-build from the console I get the following:
”’ndk-build’ is not recognized as an internal or external command,
operable program or batch file.
D:\Development\workspace\android-studio\tess-two-master\tess-two>
Hi, I’m getting an error when I run the command “ant release”. I’ve added JAVA_HOME variable and set its value as the path to the installation of the JDK.
whenever i use “android update project –target 1 –path C:\tess\tess-two” it always shows an error. i.e., android is not recognized as an internal or external.
Please help me as soon as possible.
I am getting an error while compiling “android update project –target 1 –path C:\tess\tess-two”.
Error is “Flag ‘-target’ is not valid for ‘update project’.” What to do next?
While running “ant release” command, i am getting an error. The error message is:
Buildfile: C:\Users\Pial-PC\Desktop\tesseract\eyes-two\build.xml
BUILD FAILED
C:\Users\Pial-PC\Desktop\tesseract\eyes-two\build.xml:46: sdk.dir is missing. Make sure to generate local.properties using ‘android update project’ or to inject it through an env var
Total time: 1 second
It helps me a lot. Thank you very much!
Thanks a lot Matz.
I am following the steps mentioned here: https://coderwall.com/p/eurvaq/tesseract-with-andoird-and-gradle
In Configure tess-two with gradle tells to copy and paste the tess-two folder to my Project but when I paste, I get an error stating Cannot create class-file and none of my `.java’ files get copied.
Where am I going wrong?Please help me
I am getting this error while building ndk-build in eyes-two
C:\Users\VAIO\ocr\tess>ndk-build
Android NDK: Could not find application project directory !
Android NDK: Please define the NDK_PROJECT_PATH variable to point to it.
C:\android-ndk-r10e\build/core/build-local.mk:143: *** Android NDK: Aborting
. Stop.
Hi Martz, I have tried to follow the step you described above. But I believe from step 10 it is confusing as lot of people stucked in that portion. It will be very much helpful if you can explain a little more from step 10.
Hi Matz,
i was doing the same as the steps mentioned. But when i am doing ndk build in eyes two i am getting some error :
Android NDK: Your APP_BUILD_SCRIPT points to an unknown file: ./jni/Android.mk
E:/android-ndk-r10e/build/core/add-application.mk:199: *** Android NDK: Aborting
… . Stop.
could you please let me know what is the issue.
Thanks.
i got some problem in step 6
Hello and thank you for sharing your experience,
I successfully got the app running but after returning from camera (taken image confirmation) got this error:
java.lang.UnsatisfiedLinkError: Couldn’t load jpgt from loader dalvik.system.PathClassLoader[DexPathList[[zip file “/data/app/com.example.myapplication-1.apk”],nativeLibraryDirectories=[/data/app-lib/com.example.myapplication-1, /vendor/lib, /system/lib]]]: findLibrary returned null
How could I get rid of that?
Thanks
Thank you very much.
Thanks a lot man, you were awesome 🙂
Hi,my Simple android ocr is working well..But i want to replace the english trained data file with my new trained data file.How can i do that?..Can someone help me please!!..Thanks in advance.
just replace eng.traindata into your train-data folder with your language data file.
Hello, I am getting UnsatisfiedLinkError in initializing the project.
05-07 12:02:30.844: E/AndroidRuntime(4477): java.lang.UnsatisfiedLinkError: dlopen failed: cannot locate symbol “rand” referenced by “liblept.so”…
Any help would b good.
Which version of the NDK and which version of tess-two are you using?
Hello, It was issue related to toolchain version of NDK. previously I was using 4.9 which was causing the error. I moved backwards to version 4.8 and the issue solved.
Can you help me on this? I got this error msg:
05-11 15:56:08.093: I/DEBUG(2233): Abort message: ‘sart/runtime/check_jni.cc:65] JNI DETECTED ERROR IN APPLICATION: JNI GetMethodID called with pending exception ‘java.lang.NoSuchFieldError’ thrown in void com.googlecode.tesseract.android.TessBaseAPI.nativeClassInit():-2′
What method were you calling when you got that error message?
Hi Robert,
I think I got the error message when calling this method: nativeClassInit();
Regards,
Djaja
Hmm, I’m having trouble reproducing the error. What version of Android are you running on? And what version of the NDK did you build with?
I am getting this error message when this method is called:
TessBaseAPI baseApi = new TessBaseAPI();
I love this tutorial it really helped me a lot.
Thanks Gautam you rock
Hello Gautam,
My OCR project build was successful.When i run it on my phone it capture the image & store in sdCard but finally popup saying “Unfortunately OCRapp has stopped working”.
Note: i haven’t import language file anywhere. Do i have to import it.
( tell me how and which file to download for English )
(where to save the language file )
Please help me very interested in your tutorial.
did you find a solution for that?
hello anushka please help me.. I compile this tess-two but when run android update project i can’t. It says android is not recognized as an internal or external. Please help me what to do. As soon as possible. Please
@Diadem
I think first you have check whether your andorid sdk path is correct if it is correct try following command to update your projects.
android update project -p .
be carefull you must enter a dot at the end of command.
@Matz,
I’m using Android studio,I followed your instructions here.But stuck setting Environmental variable for windows. Do i have to use Cygwin. Because I install ndk then in the 8th instruction commandline says “android is not recognized as external or internal command.
Help me on this.
Hello!
Did you found a way through that? I am having the same problem :\
Thank you
I am also having the same problem,Did you find a way through that?
Thank you
i’m so late, but for the others who want a answers,
“android update project” command come from the Android SDK, not from NDK, i had the same problem.
Android SDK from Android Studio is in AppData directory.
C:\Users\Me\AppData\Local\Android\sdk\tools\Android.bat
My Android sdk in not in environment variable.
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project –t 1 –p C:\Users\Me\Downloads\tess\tess_two
or
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project –-target 1 –-path C:\Users\Me\Downloads\tess\tess_two
Thanks a lot….. Very useful blog…..
06-23 05:46:25.192: D/dalvikvm(1420): Added shared lib libjavacore.so 0x0
06-23 05:46:25.212: D/dalvikvm(1420): Trying to load lib libnativehelper.so 0x0
06-23 05:46:25.212: D/dalvikvm(1420): Added shared lib libnativehelper.so 0x0
06-23 05:46:25.212: D/dalvikvm(1420): No JNI_OnLoad found in libnativehelper.so 0x0, skipping init
06-23 05:46:25.432: D/dalvikvm(1420): Note: class Landroid/app/ActivityManagerNative; has 179 unimplemented (abstract) methods
06-23 05:46:25.962: E/memtrack(1420): Couldn’t load memtrack module (No such file or directory)
06-23 05:46:25.962: E/android.os.Debug(1420): failed to load memtrack module: -2
06-23 05:46:26.252: D/AndroidRuntime(1420): Calling main entry com.android.commands.pm.Pm
06-23 05:46:26.322: W/ActivityManager(385): No content provider found for permission revoke: file:///data/local/tmp/OCRTest.apk
06-23 05:46:26.342: E/Vold(51): Error creating imagefile (Read-only file system)
06-23 05:46:26.342: E/Vold(51): ASEC image file creation failed (Read-only file system)
06-23 05:46:26.342: W/Vold(51): Returning OperationFailed – no handler for errno 30
06-23 05:46:26.352: E/PackageHelper(644): Failed to create secure container smdl2tmp1
06-23 05:46:26.352: E/DefContainer(644): Failed to create container smdl2tmp1
06-23 05:46:26.352: W/ActivityManager(385): No content provider found for permission revoke: file:///data/local/tmp/OCRTest.apk
06-23 05:46:26.442: D/dalvikvm(385): GC_EXPLICIT freed 452K, 30% free 5735K/8136K, paused 6ms+7ms, total 89ms
06-23 05:46:26.482: D/AndroidRuntime(1420): Shutting down VM
06-23 05:46:26.482: D/dalvikvm(1420): Debugger has detached; object registry had 1 entries
06-23 05:46:26.492: D/dalvikvm(1420): Compiler shutdown in progress – discarding request
My code gives this error boss help me with it
06-24 14:29:11.398: E/AndroidRuntime(32082): FATAL EXCEPTION: main
06-24 14:29:11.398: E/AndroidRuntime(32082): Process: com.example.asd, PID: 32082
06-24 14:29:11.398: E/AndroidRuntime(32082): java.lang.UnsatisfiedLinkError: dalvik.system.PathClassLoader[DexPathList[[zip file “/data/app/com.example.asd-2/base.apk”],nativeLibraryDirectories=[/vendor/lib, /system/lib]]] couldn’t find “liblept.so”
06-24 14:29:11.398: E/AndroidRuntime(32082): at java.lang.Runtime.loadLibrary(Runtime.java:366)
06-24 14:29:11.398: E/AndroidRuntime(32082): at java.lang.System.loadLibrary(System.java:988)
06-24 14:29:11.398: E/AndroidRuntime(32082): at com.googlecode.tesseract.android.TessBaseAPI.(TessBaseAPI.java:43)
06-24 14:29:11.398: E/AndroidRuntime(32082): at com.example.asd.MainActivity.onCreate(MainActivity.java:24)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.Activity.performCreate(Activity.java:5990)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1106)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2278)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2387)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.ActivityThread.access$800(ActivityThread.java:151)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1303)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.os.Handler.dispatchMessage(Handler.java:102)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.os.Looper.loop(Looper.java:135)
06-24 14:29:11.398: E/AndroidRuntime(32082): at android.app.ActivityThread.main(ActivityThread.java:5254)
06-24 14:29:11.398: E/AndroidRuntime(32082): at java.lang.reflect.Method.invoke(Native Method)
06-24 14:29:11.398: E/AndroidRuntime(32082): at java.lang.reflect.Method.invoke(Method.java:372)
06-24 14:29:11.398: E/AndroidRuntime(32082): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:903)
06-24 14:29:11.398: E/AndroidRuntime(32082): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:698)
Hi. I’m trying to use it in Eclipse but nothing is recognized. In particular, I have some troubles with “import android.graphics.Color;” where “The import android cannot be resolved” can you help me?
I am in a big trouble. Unable to add the jni, as i am completely new to JNI. plus the application crashes the moment i click on scan.
Please help me Gautam.
hi gautam,
i was able to successfully run the app in genymotion, and was trying to play with OCR using the laptop webcam. it is throwing different characters.
clif please help me in android update project error code.
I need help. Please revert.
New to OCR want to read My ID card with this but its not giving me expected result. Please let me know how i can define Region of Inetrest like left|Right|Bottom|top etc or Coordinates ??????
how can i crop something out of the bitmap to be analized individual ?
hi. i want to develop a project for analysing text from form which is user entered. So, i want to scan that form and convert that image into text using ocr concept. i want to do it in android app. i am new to android app development and i am using eclipse. So, can you tell the step by step process for creating ocr application clearly? because, i don’t know how to use api. So, can you help me?
Hi, I followed the tutorial but adapted it for Android Studio. The app loaded ok then I took a photo of text and when I tap on the check mark the app crashed. The first error message I got was:
… failed: dlopen failed: cannot locate symbol “png_set_longjmp_fn” referenced by “liblept.so”…
There are some other errors but I think they may be related to the first one. Can anyone help me to solve his problem?
Thanks if you can point me in the right direction.
I have made a very efficient and easy to use OCR library compatible with gradle and Android Studio.
https://github.com/priyankvex/Easy-Ocr-Scanner-Android
If you guys can use it and review it. That would be great.
Priyank Verma, I was able to get your built in “app” to work. It’s probably going to take me a couple of days to figure out how to make it work with mine (I’m new to this). Just wanted to say thanks!
Got it to work in my app. Thanks again Priyank!
Hi Priyanka,
Can you please help me to integrate it in cordova project?
Thanks in advance!
I am getting this error while building the files java.lang.UnsatisfiedLinkError: Couldn’t load pngt: findLibrary returned null . Can anybody help ??
Are you using the latest version of tess-two? Are you using a precompiled library, or did you do an ndk-build on the latest version yourself?
Hi!,
I have cloned the repository then the following:
– added to path:
*export PATH=$PATH:/mnt/sdb1/android-sdk-linux
*export PATH=$PATH:/mnt/sdb1/android-sdk-linux/platform-tools
*export PATH=$PATH:/mnt/sdb1/android-ndk-r10e
ndk-build and I get the following error:
Android NDK: ERROR:/mnt/sdb1/android-ndk-r10e/sources/cxx-stl/gnu-libstdc++/Android.mk:gnustl_static: LOCAL_SRC_FILES points to a missing file
Android NDK: Check that /mnt/sdb1/android-ndk-r10e/sources/cxx-stl/gnu-libstdc++/4.8/libs/armeabi-v7a/thumb/libgnustl_static.a exists or that its path is correct
/mnt/sdb1/android-ndk-r10e/build/core/prebuilt-library.mk:45: *** Android NDK: Aborting . Stop.
Please advise what to do. I’m running FedoraCore 21.
Does that libgnustl_static.a file exist in that directory?
Yes, it is.
hi we are trying to recognise hindi language using the hin.trainedata available for tesseract but it is not recognizing anything but when we replace the hindi training data by english it recognizes everything . any suggestion what might be the cause thank you
You didn’t leave enough details. Did you also change your init() from “eng” to “hin”?
Even I tried this Tesseract OCR part in Android. I was facing a run time error stating :
java.lang.UnsatisfiedLinkError: dlopen failed: cannot locate symbol “png_set_longjmp_fn” referenced by “liblept.so”
I tried many things to figure it out. But ultimately replacing the libpng folder ( source ) with the one in this repo ( https://github.com/julienr/libpng-android ) and building it again ( ndk-build ) helped me. The app now runs without any error. Thought of sharing this. 🙂
Are you using the latest code? Would you please open up an issue on the tess-two project with some steps to reproduce the error?
No I didn’t. I think in the latest commit the bug is fixed. Sorry, didn’t look into that.
Gautam, I keep getting java.noclassdef found error wen I type ant release..am running windows 7
I am trying to make a software which can extract the MICR from the check. I am taking the screenshot from the camera and try to read the routing number and check number at the bottom of the check. But not able to read it accurately. Anyone please suggest what to do.
Hi Gautam
Your tutorial work fine but I want to ask that for a single character can i get various output with their percentage.
Waiting for your reply.
Hi Gautam,Thank you for sharing this tutorial.I want to get the focus for the camera to select a text in the image as you shown in the screenshot above..how can i achieve that.can you please suggest.
Hi Devika,
Did you find how to set the focus? I am too stuck here.
When i try to compile the project i got this Error.
C:\Users\Sol\AndroidStudioProjects\MOBCharge\tesstwo\src\main\jni\com_googlecode_leptonica_android\common.h:22:24: fatal error: allheaders.h: No such file or directory
#include
^
compilation terminated.
make.exe: *** [C:\Users\Sol\AndroidStudioProjects\MOBCharge\tesstwo\build\intermediates\ndk\release\obj/local/arm64-v8a/objs/tesstwo/C_\Users\Sol\AndroidStudioProjects\MOBCharge\tesstwo\src\main\jni\com_googlecode_leptonica_android\box.o] Error 1
Error:Execution failed for task ‘:tesstwo:compileReleaseNdk’.
> com.android.ide.common.process.ProcessException: org.gradle.process.internal.ExecException: Process ‘command ‘C:\android-ndk-r10e\ndk-build.cmd” finished with non-zero exit value 2
hi , in the application of test OCR it if I choose the Arabic ocr it should download the training data every time I open the app .
it don’t make the same in the other languages .
First its a great guide.
I am trying to implement the same currently, and i have run into small problem. I want to execute this project, please help me out here.
So my problem is like this:-
I am using windows 7 pc, android studio.
so am following the instructions as given here
https://gaut.am/making-an-ocr-android-app-using-tesseract/#comment-184181
When i try to implment pint no 8 (adding path), i get following error.
C:\Users\mack\AndroidStudioProjects\tess-two-master\tess-two-master\tess-two>android update project -target 1 -path C:\Users\mack\AndroidStudioProjects\tess-two-master\tess-two-maste
r\tess-two
Error: Argument ‘C:\Users\mack\AndroidStudioProjects\tess-two-master\tess-two-master\tess-two’ is not recognized.
Usage:
android [global options] update project [action options]
Global options:
-s –silent : Silent mode, shows errors only.
-v –verbose : Verbose mode, shows errors, warnings and all messages.
–clear-cache: Clear the SDK Manager repository manifest cache.
-h –help : Help on a specific command.
Action “update project”:
Updates an Android project (must already have an AndroidManifest.xml).
Options:
-s –subprojects: Also updates any projects in sub-folders, such as test
projects.
-l –library : Directory of an Android library to add, relative to this
project’s directory.
-p –path : The project’s directory. [required]
-n –name : Project name.
-t –target : Target ID to set for the project.
Please help me out here, i tried to google the error but didnt find anything fruitful.
I tried this :
my sdk is not in environment variable.
my ndk is not too.
path of my eyes-two : C:\Users\Me\Downloads\tess\eyes-two
command in eyes-two directory :
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project –target 1 –path C:\Users\Me\Downloads\tess\tess_two
And it works :
Result displayed:
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project -t 1 -p C:\Users\Me\Downloads\tess\tess-two
updated project.properties
updated local.properties
Added file C:\Users\Me\Downloads\tess\tess-two\proguard-project.txt
oups mistake, command in eyes-two directory:
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project -–target 1 –-path C:\Users\Me\Downloads\tess\tess_two
or
C:\Users\Me\Downloads\tess\eyes-two>C:\Users\Me\AppData\Local\Android\sdk\tools\android update project –t 1 –p C:\Users\Me\Downloads\tess\tess_two
action options synthax
-p or –path
-t or –target
great tutorial. still works like a charm. our further intention is to further train the model to recognize handwriting better, any idea how that can be done?
Thank you.
01-29 14:20:53.779: W/dalvikvm(25363): Exception Ljava/lang/UnsatisfiedLinkError; thrown while initializing Lcom/googlecode/tesseract/android/TessBaseAPI;
01-29 14:20:53.779: D/AndroidRuntime(25363): Shutting down VM
...
01-29 14:20:53.781: E/AndroidRuntime(25363): at dalvik.system.NativeStart.main(Native Method)
it occurs “unfortunately stopped” error after capturing the image
i have same problem . what am i doiiing???
brother i made app successfully but its not giving the right result all the time kindly tell me how to improve accuracy
I am using windows 8.1 pro with android studio 1.51 ,I am getting an error in step 9 ,it says that ant is not recognized as an internal or external command , can someone please help me out , thanks in advance
I’m using a Nexus 6p for testing and every time I press the tick after I take a picture, it returns a 0 result code, meaning the onPhotoTaken method never get’s hit. Any ideas as to why it always returns 0?
hello Follow all steps but
i have problem in my app ,
“unfortunately stopped” error after capturing the image and exit my app
please help me
Good job, we need people like you
thanx.
But my story is that i can’t be able to compile on my android device it could not be able to resolve the native function path.
will i get some error if i try this in android studio ? TY 🙂
Hi guys, can i konw how photomath app is working… Am doing it as my final year project. Can any one help me in developing it
Thank you. your tutorial very help me create ocr for my application in android
did you applied it in android studio.if yes then please contact me at aimensyed528@gmail.com
hello friends imgetting step 7) Write “ndk-build” and wait.
C:\Users\admin\Downloads\tess-two-master\eyes-two>ndk-build
Android NDK: Your APP_BUILD_SCRIPT points to an unknown file: ./jni/Android.mk
C:/android-ndk-r11c/build//../build/core/add-application.mk:195: *** Android NDK
: Aborting… . Stop.
C:\Users\admin\Downloads\tess-two-master\eyes-two>
Please help
Hi,
I fixed this problem with copy “tess/eyes-two/src/main/*” to “tess/eyes-two/*” (you need to override the “AndroidManifest” file.
edit the “tess/eyes-two/jni/Android.mk” :
line 2 for:
TESSERACT_TOOLS_PATH := $(call my-dir)/../../tess-two
(remove some ../../)
and it should be good
Thank you Jean-philippe emond,
I had the same issue Surender had and your reply fixed it.
hello friends im getting error step 8) also
C:\Users\admin\Downloads\tess-two-master\eyes-two>C:\Users\admin\AppData\Local\A
ndroid\sdk\tools\android update project -t 1 -p C:\Users\admin\Downloads\tess-tw
o-master\tess-two
Error: Argument ‘C:\Users\admin\Downloads\tess-two-master\tess-two’ is not recog
nized.
Surender, did you resolve this error? If so, how?
Hi!. How can use this librari with Android Studio?.
I ‘m trying to use the library , but do not know how is used.
To everyone having trouble: Please take a look at the updated Usage instructions in the tess-two project Readme. The project is much easier to use now, and you no longer have to do the ndk-build step yourself!
hello im umer …how can we do this if we have to read text from two different parts of a picture at the same time ? for example reading 1) meter no and 2) units from electricity meter
Error Tesseract-OCR Cannot resolve corresponding JNI function)
I am using tesseract with android studio. I want to increase word scan accuracy. May i know how can i achieve this? I have used below link for demo.
https://github.com/priyankvex/Easy-Ocr-Scanner-Android
I followed your step and I can build tess two in cmd with the command “ndk-build”. But I can’t write another command which is – ” android update project –target 1 –path C:\tess\tess-two “. However I did it only says – ” ‘android’ is not recognized as an internal or external command, operable program or batch file. ” Is there anything I have misunderstood about your post? Please tell me where did I go wrong.
Why I can’t write ‘ant release’ . It always show ‘ant’ is recognize as an internal or external command,operable program or batch file.
I add my system environment variables as I did in step 2 and ADD a new variable with name “JAVA_HOME” and value the path to my jdk but It always show that. Please help me
I am using android studios IDE.Can you help me with this error “Could not initialize Tesseract API with language=eng!”.
hello, i am working on a project on android application which extract receipt images text and save it using OCR API. The problem i am having is that i want some way so that image potion can be selectable to user so that it highlight amount and date on the receipt and it will be extracted in separate text box. I want this because i am having accuracy problem with java regex.kindly guide me any way so that user selects amount or date potions on the image either marking or highlighting it and ocr will extract that parts.
I have doubt regarding security. Will my data be revealed to outside other than my app. I mean, will it stored or sent to some third party for processing. Can I make sure that my Image information or text inside that is not sent any third party without my control.
Good morning everyone!
Is it possible to have two areas for taking the picture?
in short, to have two focusboxview ?
thank you
Does the data collected from the image need to be sent through to tesseract, or can it be built in such a way that the information isn’t sent away anywhere?
OCR Quickly – Text Scanner – https://play.google.com/store/apps/details?id=com.urysoft.ocrquickly
Added to the post! 🙂
in the pass 9, if it complains, execute the next command line for pass the project to target 8:
android update project –subprojects –path . –target “android-8”
It work for me
how to support portrait mode?
I had done the portrait mode support , but the recognition was very poor.
Where should i type this step “Build this project using these commands (here, tess-two is the directory inside tess-two – the one at the same level as of tess-two-test):
cd /tess-two
ndk-build
android update project –path .
ant release”
Hey Gautam, i followed all the instructions here and it work, but the app doesn’t give an accurate result. All I’m getting is weird text that doesn’t belong to the image even if I binarized and remove noise on the image. . .
Can u give us a suggestion? Please. . . . . 🙂
I have got to the stage to type in ‘ant release’ but when i do, i get an error message saying, ” ‘ant’ is not recognised as an internal or external command” but I’ve added an environment variable JAVA_HOME with the correct directory of the jdk. What have i done wrong?
Hello, did anyone find a solution for “the app has stopped working” problem ? when I click the button to start scanning, the app crashes in my Genymotion emulator. I have tried various devices and restarted both Genymotion and Android Studio multiple times just to make sure but I have the same issue.
Please tell how may i download language file and where i have to keep it in my program…
Hey Gautam Great Tutorial 🙂 I have cloned the downloaded the applications on github but when I run the application I get this error
Error:Execution failed for task ‘:OCRTest:transformNative_libsWithStripDebugSymbolForDebug’.
> java.lang.NullPointerException (no error message)
Any ideas on what the issue is? feedback would be very appreciated 🙂
Hi Gautam! I tried using the application. It is pretty good. One thing i have found is that when i focus the images from camera on an object and then it gives me an error like
12-24 16:33:45.709: A/libc(16592): Fatal signal 11 (SIGSEGV), code 1, fault addr 0x0 in tid 16592 (roid.ocr.simple)
Also when i test the applications more than one two times adjusting the focus it gives me nothing the the recognized. The program stops after this
12-24 16:33:45.184: I/Tesseract(native)(16592): Initialized Tesseract API with language=eng
Please help!
Hello. I scan Letter “A” and already display in OcrTextView but I need to use if else for my codes. Example.
If (OCRResult == “A”){
\\do something
}
The result is always false. Whats wrong?
Hello..
I want to ask, What is tesseract can recognition Script Batak Toba?
C:\tess\tess-two>ndk-build
‘ndk-build’ is not recognized as an internal or external command,
operable program or batch file.
Hello Gautam! I am getting error…Cannot initialize camera. Please help me in this.
Hi,
It is really nice work to I want to do the same thing on IOS using teserect and openCV to detect specific text.
Could you please send me some links or an example app to guide?
Thanks in advance.
Hello Gautam,
It is an interesting article. I am doing a project which requires to read Australian IDs and Arabic IDs and convert them to text in relevant fields. Could you please help me in developing an OCR software which will be embedded in to my software. Please note I need it work offline and don’t want to pay on going license fee. One off development cost is OK.
Thanks.
Hi,
I’m facing the similar problem with offline Arabic text OCR. Did you succeed with your app?
Please let me know to edrokov@gmail.com.
Hello, I want to Reject when an image is not clear for this what can I used please guess me some filters.
Hello Gautam,
Can we use this app for text extraction from devnagiri and other languages as well if use the other traindata file?
we got this error.please reply as early as possible.
”’ndk-build’ is not recognized as an internal or external command,
operable program or batch file.
Just add ndk-path to your environment variables.
In my case I edit the “path” variable name in mine and put this:
“C:\Users\Valerie\AppData\Local\Android\sdk;” . Just make sure to put “;” at the end then restart your pc and try again running ndk-build command.
Hi, Have you tried developing a Passport MRZ scanner in Android app
how can I build this project in android studio?
Hi, I have followed all the steps correctly multiple times but I keep on getting the errors below while running the ant release command. Can someone please help me regarding this errors? Thank you/
-compile:
[javac] Compiling 24 source files to D:\tess\tess-two\bin\classes
[javac] warning: [options] source value 1.5 is obsolete and will be removed
in a future release
[javac] warning: [options] target value 1.5 is obsolete and will be removed
in a future release
[javac] warning: [options] To suppress warnings about obsolete options, use
-Xlint:-options.
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pix.java:20: e
rror: package android.support.annotation does not exist
[javac] import android.support.annotation.ColorInt;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pix.java:21: e
rror: package android.support.annotation does not exist
[javac] import android.support.annotation.Size;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Binarize.java:
19: error: package android.support.annotation does not exist
[javac] import android.support.annotation.FloatRange;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Box.java:20: e
rror: package android.support.annotation does not exist
[javac] import android.support.annotation.Size;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Boxa.java:20:
error: package android.support.annotation does not exist
[javac] import android.support.annotation.Size;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Constants.java
:19: error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntDef;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Constants.java
:56: error: cannot find symbol
[javac] @IntDef({L_INSERT, L_COPY, L_CLONE})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class Constants
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Constants.java
:79: error: cannot find symbol
[javac] @IntDef({L_SORT_INCREASING, L_SORT_DECREASING})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class Constants
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Constants.java
:89: error: cannot find symbol
[javac] @IntDef({L_SORT_BY_X, L_SORT_BY_Y, L_SORT_BY_WIDTH, L_SORT_BY_HE
IGHT, L_SORT_BY_MIN_DIMENSION,
[javac] ^
[javac] symbol: class IntDef
[javac] location: class Constants
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Edge.java:19:
error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntDef;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Edge.java:38:
error: cannot find symbol
[javac] @IntDef({L_HORIZONTAL_EDGES, L_VERTICAL_EDGES, L_ALL_EDGES})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class Edge
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\JpegIO.java:21
: error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntRange;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\MorphApp.java:
19: error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntDef;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\MorphApp.java:
38: error: cannot find symbol
[javac] @IntDef({L_TOPHAT_BLACK, L_TOPHAT_WHITE})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class MorphApp
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pixa.java:20:
error: package android.support.annotation does not exist
[javac] import android.support.annotation.Size;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Rotate.java:19
: error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntRange;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:22: error: package android.support.annotation does not exist
[javac] import android.support.annotation.IntDef;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:23: error: package android.support.annotation does not exist
[javac] import android.support.annotation.WorkerThread;
[javac] ^
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:60: error: cannot find symbol
[javac] @IntDef({PSM_OSD_ONLY, PSM_AUTO_OSD, PSM_AUTO_ONLY, PSM_AUTO
, PSM_SINGLE_COLUMN,
[javac] ^
[javac] symbol: class IntDef
[javac] location: class PageSegMode
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:124: error: cannot find symbol
[javac] @IntDef({OEM_TESSERACT_ONLY, OEM_CUBE_ONLY, OEM_TESSERACT_CUBE_C
OMBINED, OEM_DEFAULT})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class TessBaseAPI
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:151: error: cannot find symbol
[javac] @IntDef({RIL_BLOCK, RIL_PARA, RIL_TEXTLINE, RIL_WORD, RIL_SY
MBOL})
[javac] ^
[javac] symbol: class IntDef
[javac] location: class PageIteratorLevel
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pix.java:130:
error: cannot find symbol
[javac] public boolean getDimensions(@Size(min=3) int[] dimensions) {
[javac] ^
[javac] symbol: class Size
[javac] location: class Pix
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pix.java:302:
error: cannot find symbol
[javac] public void setPixel(int x, int y, @ColorInt int color) {
[javac] ^
[javac] symbol: class ColorInt
[javac] location: class Pix
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Binarize.java:
124: error: cannot find symbol
[javac] @FloatRange(from=0.0, to
=1.0) float scoreFraction) {
[javac] ^
[javac] symbol: class FloatRange
[javac] location: class Binarize
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Binarize.java:
190: error: cannot find symbol
[javac] public static Pix sauvolaBinarizeTiled(Pix pixs, int whsize, @Fl
oatRange(from=0.0) float factor,
[javac] ^
[javac] symbol: class FloatRange
[javac] location: class Binarize
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Box.java:190:
error: cannot find symbol
[javac] public boolean getGeometry(@Size(min=4) int[] geometry) {
[javac] ^
[javac] symbol: class Size
[javac] location: class Box
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Boxa.java:121:
error: cannot find symbol
[javac] public boolean getGeometry(int index, @Size(min=4) int[] geometr
y) {
[javac] ^
[javac] symbol: class Size
[javac] location: class Boxa
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\JpegIO.java:64
: error: cannot find symbol
[javac] public static byte[] compressToJpeg(Pix pixs, @IntRange(from=0,
to=100) int quality,
[javac] ^
[javac] symbol: class IntRange
[javac] location: class JpegIO
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Pixa.java:359:
error: cannot find symbol
[javac] public boolean getBoxGeometry(int index, @Size(min=4) int[] dime
nsions) {
[javac] ^
[javac] symbol: class Size
[javac] location: class Pixa
[javac] D:\tess\tess-two\src\com\googlecode\leptonica\android\Rotate.java:10
6: error: cannot find symbol
[javac] public static Pix rotateOrth(Pix pixs, @IntRange(from=0, to=3) i
nt quads) {
[javac] ^
[javac] symbol: class IntRange
[javac] location: class Rotate
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:508: error: cannot find symbol
[javac] @WorkerThread
[javac] ^
...
[javac] @WorkerThread
[javac] ^
[javac] symbol: class WorkerThread
[javac] location: class TessBaseAPI
[javac] D:\tess\tess-two\src\com\googlecode\tesseract\android\TessBaseAPI.ja
va:753: error: cannot find symbol
[javac] @WorkerThread
[javac] ^
[javac] symbol: class WorkerThread
[javac] location: class TessBaseAPI
[javac] 36 errors
[javac] 3 warnings
BUILD FAILED
C:\Users\Valerie\AppData\Local\Android\sdk\tools\ant\build.xml:716: The followin
g error occurred while executing this line:
C:\Users\Valerie\AppData\Local\Android\sdk\tools\ant\build.xml:730: Compile fail
ed; see the compiler error output for details.
Total time: 18 seconds
facing Same Error..any solution for it ?
Hi,
I saw now it’s working just for English language. How to make it to work for all languages?
I want to detect Marathi/Hindi language and get it translated in English. How to do the same?
I am using tess two 9.0.0 to scan MRZ of passport. Its working fine but if used continuously the ‘ getHOCRText ‘ method become very slow to read the text. To improve the performance I am passing the binarised image but not getting any result. Can’t figure out the issue. Kindly help.
Hi Gautam
I am developing same app on iOS Please tell me what should be folder structure. I have done 1 for Camera and one for OCR is it correct? As I always get error of -h file missing.
Hey nice article but I was searching a way to call this code from c++ on android is that possible